]编写一个 C++ 程序,实现一个简单的银行账户类 BankAccount,包含账号、余额的成员变量,并实现存款和取款的功能。要求实现构造函数、析构函数,以及用于显示账户信息的函数。
时间: 2024-10-01 22:07:41 浏览: 69
在C++中,我们可以创建一个名为BankAccount的类来模拟一个基本的银行账户,以下是这个类的简单实现:
```cpp
#include <iostream>
class BankAccount {
private:
int accountNumber; // 账号
double balance; // 余额
public:
// 构造函数
BankAccount(int number) {
accountNumber = number;
balance = 0.0; // 初始余额为0
std::cout << "Account created with number " << accountNumber << std::endl;
}
// 存款功能
void deposit(double amount) {
if (amount > 0) {
balance += amount;
std::cout << "Deposited: $" << amount << ". New balance: $" << balance << std::endl;
} else {
std::cout << "Invalid deposit amount." << std::endl;
}
}
// 取款功能
bool withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
std::cout << "Withdrew: $" << amount << ". New balance: $" << balance << std::endl;
return true;
} else {
std::cout << "Insufficient funds for withdrawal." << std::endl;
return false;
}
}
// 显示账户信息函数
void displayInfo() const {
std::cout << "Account Number: " << accountNumber << std::endl;
std::cout << "Balance: $" << balance << std::endl;
}
~BankAccount() {
std::cout << "Account destroyed: " << accountNumber << std::endl;
}
};
int main() {
BankAccount myAccount(123456);
myAccount.deposit(1000.00);
myAccount.withdraw(500.00);
myAccount.displayInfo();
return 0;
}
```
在这个例子中,`BankAccount`类包含了私有成员变量accountNumber和balance,以及对应的公共方法deposit, withdraw和displayInfo。构造函数接受一个账号作为参数,而析构函数会在对象生命周期结束时自动调用,显示账户被销毁的信息。
在main函数中,我们创建了一个BankAccount对象并进行了存款和取款操作,最后展示了账户信息。
阅读全文
相关推荐
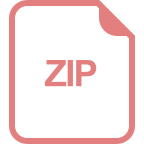
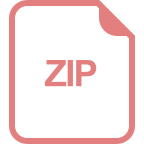
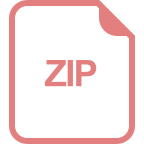




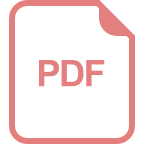
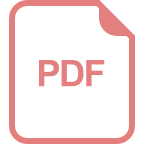
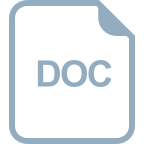
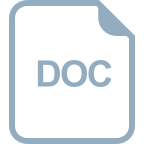
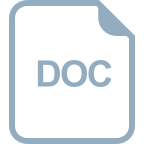
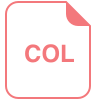
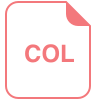




