TableView中currentRow的使用
时间: 2024-04-22 20:26:13 浏览: 14
在 TableView 中,currentRow 是一个属性,用于获取当前选中行的索引。它表示当前用户选中的行在表格中的位置。
你可以使用以下方式来使用 currentRow 属性:
```cpp
int currentRow = tableView->currentRow();
```
这将返回当前选中行的索引值,如果没有选中任何行,它将返回 -1。
请注意,currentRow 属性适用于具有单选或多选功能的 TableView。如果 TableView 不允许选择行或只允许选择单个行,则 currentRow 属性可能不适用。
希望这个回答能够帮到你!如果你还有其他问题,请随时提问。
相关问题
qt中tableview复制粘贴
在Qt中,可以使用QTableView类来创建一个表格视图。如果你想在表格视图中实现复制粘贴功能,可以通过以下步骤来实现:
1. 在你的QTableView类中,添加以下代码:
```
QShortcut *copyShortcut = new QShortcut(QKeySequence::Copy, this);
connect(copyShortcut, &QShortcut::activated, this, &MyTableView::copy);
QShortcut *pasteShortcut = new QShortcut(QKeySequence::Paste, this);
connect(pasteShortcut, &QShortcut::activated, this, &MyTableView::paste);
```
这里使用了QShortcut类来创建复制和粘贴的快捷键,并将其连接到copy()和paste()槽函数。
2. 实现copy()和paste()槽函数:
```
void MyTableView::copy()
{
QModelIndexList selectedIndexes = selectionModel()->selectedIndexes();
if (selectedIndexes.isEmpty())
return;
qSort(selectedIndexes);
QString text;
int currentRow = selectedIndexes.first().row();
foreach (const QModelIndex &index, selectedIndexes) {
if (index.row() != currentRow) {
text += '\n';
currentRow = index.row();
} else if (text.length() > 0) {
text += '\t';
}
text += index.data(Qt::DisplayRole).toString();
}
QClipboard *clipboard = QApplication::clipboard();
clipboard->setText(text);
}
void MyTableView::paste()
{
QClipboard *clipboard = QApplication::clipboard();
QString text = clipboard->text();
QModelIndexList selectedIndexes = selectionModel()->selectedIndexes();
if (selectedIndexes.isEmpty())
return;
qSort(selectedIndexes);
int row = selectedIndexes.first().row();
int col = selectedIndexes.first().column();
QStringList lines = text.split('\n');
foreach (const QString &line, lines) {
QStringList fields = line.split('\t');
foreach (const QString &field, fields) {
QModelIndex index = model()->index(row, col);
model()->setData(index, field, Qt::EditRole);
col++;
}
row++;
col = selectedIndexes.first().column();
}
}
```
在copy()函数中,我们首先获取当前选中的单元格的索引,然后按行列顺序遍历所有选中的单元格,并将其内容复制到一个QString变量中。最后,将复制的文本设置到剪贴板上。
在paste()函数中,我们首先从剪贴板获取文本,并将其按行列分割成单元格内容。然后,遍历选中的单元格,将剪贴板中的文本依次粘贴到每个单元格中。
3. 最后,在你的QTableView类中添加以下头文件:
```
#include <QShortcut>
#include <QApplication>
#include <QClipboard>
```
这些头文件包含了我们需要使用的类和函数。
在QT中获取tableview当前选中行
在QT中获取TableView当前选中行,可以通过以下代码实现:
```cpp
QModelIndexList selectedRows = tableView->selectionModel()->selectedRows();
if(!selectedRows.empty()){
int currentRow = selectedRows.at(0).row();
}
```
首先,我们使用`selectionModel()`方法获取TableView的选择模型。然后,我们使用`selectedRows()`方法获取选中的行的模型索引列表。最后,我们可以通过`row()`方法获取选中行的行号。
请注意,`selectedRows()`方法返回的是一个列表,因此我们可以使用`empty()`方法检查列表是否为空,以免出现空指针异常。
相关推荐







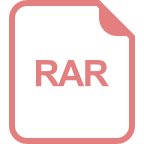
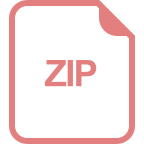
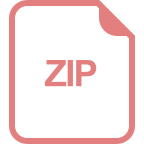