数据结构循环单链表C语言上机操作
时间: 2024-06-14 09:02:19 浏览: 247
循环单链表是链表的一种特殊形式,它的特点是链表中的最后一个节点的指针指向第一个节点,形成一个闭合的环。在C语言中,操作循环单链表通常涉及创建、插入、删除和遍历等基本操作。
以下是一些基本操作的C语言实现概览:
1. **创建循环链表**:
- 定义节点结构体,包含数据域和指向下一个节点的指针(如果是在头结点的最后一个节点,指针会指向自身)。
- 创建链表时,可以初始化一个头节点,然后递归地添加新节点到链尾。
```c
struct Node {
int data;
struct Node* next;
};
// 创建循环链表
void createCircularList(struct Node** head, int size, ...) {
...
}
```
2. **插入节点**:
- 可以在链表的头部、尾部或指定位置插入节点。
- 在头部插入,更新头节点的next;在尾部插入,找到当前尾节点,然后更新其next指向新节点,并将新节点next指向头节点。
```c
void insertNode(struct Node** head, int data, insertionPosition) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = (insertionPosition == 1) ? head : (*(head)->next);
// 如果在尾部插入,更新尾节点和头节点的next指针
if (insertionPosition == 0) {
(*head)->next = newNode;
}
}
```
3. **删除节点**:
- 删除某个节点时,需要找到前一个节点,然后更新其next指针跳过要删除的节点。
- 在循环链表中删除特定位置的节点需要特别处理头节点的情况。
```c
void deleteNode(struct Node** head, int position) {
if (position == 1) { // 删除头节点
struct Node* temp = head->next;
*head = temp;
free(head);
} else {
struct Node* current = *head, *previous = NULL;
for (int i = 1; i < position && current != NULL; ++i) {
previous = current;
current = current->next;
}
if (current == NULL) return; // 未找到节点
previous->next = current->next;
}
}
```
4. **遍历循环链表**:
- 使用while循环,每次迭代都更新当前节点指向下一个节点,直到遇到第一个节点。
```c
void printList(struct Node* head) {
struct Node* temp = head;
do {
printf("%d ", temp->data);
temp = temp->next;
} while (temp != head);
}
```
阅读全文
相关推荐
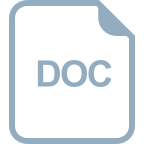
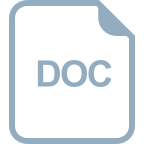
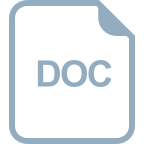

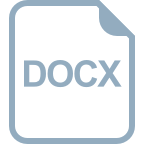
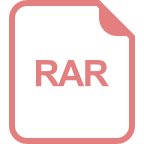
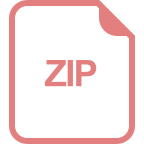
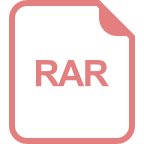
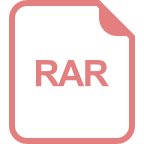
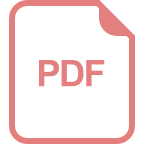
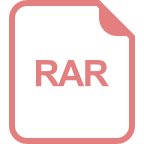
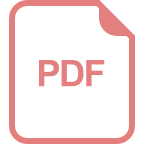
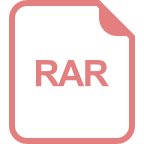
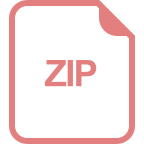
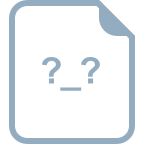
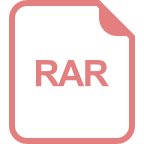
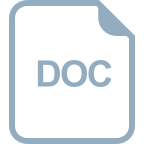
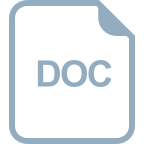