DDPG模块python
时间: 2023-11-09 13:01:08 浏览: 127
DDPG是一种深度强化学习算法,用于解决连续动作空间的问题。在Python中,可以使用TensorFlow或PyTorch等深度学习框架来实现DDPG模块。以下是一个使用PyTorch实现DDPG的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
class Actor(nn.Module):
def __init__(self, state_dim, action_dim, max_action):
super(Actor, self).__init__()
self.layer1 = nn.Linear(state_dim, 400)
self.layer2 = nn.Linear(400, 300)
self.layer3 = nn.Linear(300, action_dim)
self.max_action = max_action
def forward(self, x):
x = torch.relu(self.layer1(x))
x = torch.relu(self.layer2(x))
x = self.max_action * torch.tanh(self.layer3(x))
return x
class Critic(nn.Module):
def __init__(self, state_dim, action_dim):
super(Critic, self).__init__()
self.layer1 = nn.Linear(state_dim + action_dim, 400)
self.layer2 = nn.Linear(400 , 300)
self.layer3 = nn.Linear(300, 1)
def forward(self, x, u):
xu = torch.cat([x, u], 1)
x = torch.relu(self.layer1(xu))
x = torch.relu(self.layer2(x))
x = self.layer3(x)
return x
class DDPG(object):
def __init__(self, state_dim, action_dim, max_action):
self.actor = Actor(state_dim, action_dim, max_action).to(device)
self.actor_target = Actor(state_dim, action_dim, max_action).to(device)
self.actor_target.load_state_dict(self.actor.state_dict())
self.actor_optimizer = optim.Adam(self.actor.parameters(), lr=1e-4)
self.critic = Critic(state_dim, action_dim).to(device)
self.critic_target = Critic(state_dim, action_dim).to(device)
self.critic_target.load_state_dict(self.critic.state_dict())
self.critic_optimizer = optim.Adam(self.critic.parameters(), lr=1e-3)
self.max_action = max_action
def select_action(self, state):
state = torch.FloatTensor(state.reshape(1, -1)).to(device)
return self.actor(state).cpu().data.numpy().flatten()
def train(self, replay_buffer, batch_size=64, discount=0.99, tau=0.005):
state, action, next_state, reward, not_done = replay_buffer.sample(batch_size)
state = torch.FloatTensor(state).to(device)
action = torch.FloatTensor(action).to(device)
next_state = torch.FloatTensor(next_state).to(device)
reward = torch.FloatTensor(reward).to(device)
not_done = torch.FloatTensor(1 - not_done).to(device)
# Update critic
next_action = self.actor_target(next_state)
target_Q = self.critic_target(next_state, next_action)
target_Q = reward + (not_done * discount * target_Q).detach()
current_Q = self.critic(state, action)
critic_loss = nn.MSELoss()(current_Q, target_Q)
self.critic_optimizer.zero_grad()
critic_loss.backward()
self.critic_optimizer.step()
# Update actor
pred_action = self.actor(state)
actor_loss = -self.critic(state, pred_action).mean()
self.actor_optimizer.zero_grad()
actor_loss.backward()
self.actor_optimizer.step()
# Update target networks
for param, target_param in zip(self.critic.parameters(), self.critic_target.parameters()):
target_param.data.copy_(tau * param.data + (1 - tau) * target_param.data)
for param, target_param in zip(self.actor.parameters(), self.actor_target.parameters()):
target_param.data.copy_(tau * param.data + (1 - tau) * target_param.data)
def save(self, filename):
torch.save(self.actor.state_dict(), filename + "_actor")
torch.save(self.critic.state_dict(), filename + "_critic")
def load(self, filename):
self.actor.load_state_dict(torch.load(filename + "_actor"))
self.critic.load_state_dict(torch.load(filename + "_critic"))
self.actor_target.load_state_dict(self.actor.state_dict())
self.critic_target.load_state_dict(self.critic.state_dict())
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
```
阅读全文
相关推荐
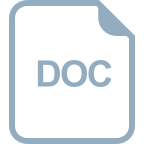
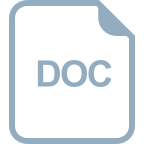
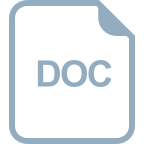
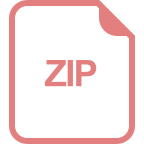
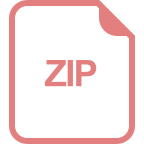
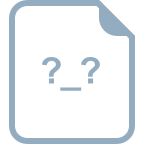
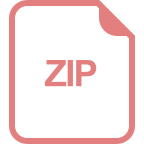
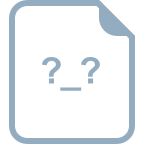
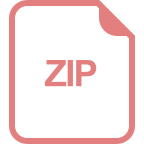
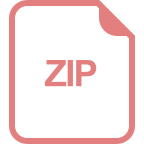
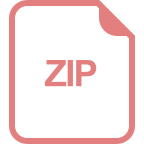
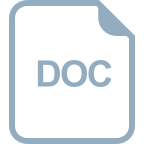
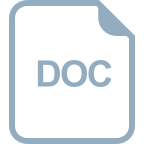
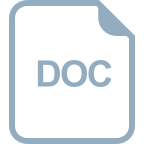
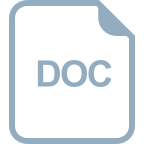
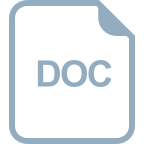
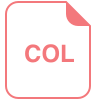
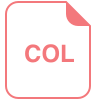
