一个更具体的Spring Boot项目示例,包括用户注册、登录、找回密码等功能
时间: 2024-10-15 16:29:20 浏览: 38
在一个典型的Spring Boot项目中,包含用户注册、登录和找回密码功能,可以创建一个基于Spring Security和Thymeleaf的简单Web应用。以下是项目的大概步骤和关键组件:
1. **设置依赖**:
- 添加Spring Web、Spring Data JPA、Spring Security和Thymeleaf依赖到`pom.xml`文件中。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<scope>runtime</scope>
</dependency>
```
2. **数据库配置**:
- 创建User实体类和对应的JPA Repository,以及数据库表结构,例如使用H2或MySQL。
3. **实体类(User)**:
```java
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
// getters and setters
}
```
4. **注册服务**:
- 实现UserService接口,提供注册方法,如`register(User user)`。
5. **登录服务**:
- 使用Spring Security的`AuthenticationManager`来处理登录验证,通过`@PostMapping("/login")`的控制器方法。
6. **密码找回**:
- 用户请求找回密码时,发送邮件验证码,然后允许用户更新密码。
7. **Thymeleaf视图**:
- 使用Thymeleaf模板来渲染HTML页面,比如注册表单、登录表单等。
8. **Controller**:
- 控制器处理HTTP请求,如`@PostMapping("/register")`处理注册,`@PostMapping("/login")`处理登录,`@GetMapping("/password/reset")`处理密码找回。
9. **安全性配置**:
- 配置Spring Security的`SecurityConfiguration`,启用认证、授权等。
**相关问题--:**
1. Spring Security如何保护API端点?
2. Thymeleaf如何在Spring Boot中集成并创建动态网页?
3. 如何在Spring Boot中实现JWT令牌验证以增强安全性?
阅读全文
相关推荐
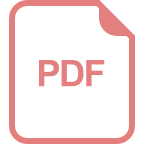
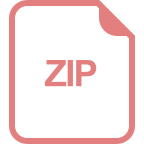
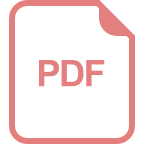
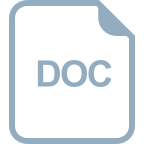
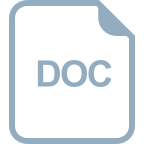
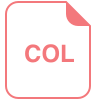
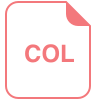











