springboot接口下载指定目录下的所有文件和文件夹
时间: 2024-11-25 15:31:39 浏览: 9
在Spring Boot应用中,如果需要提供一个API来允许用户下载指定目录下的所有文件和文件夹,通常会涉及到HTTP服务端的资源访问和文件操作。这可以通过Spring MVC和`@RestController`控制器以及`ResponseEntity`类来实现。以下是一个简单的示例:
```java
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
@RestController
public class FileDownloadController {
@GetMapping("/download/{path}")
public ResponseEntity<MultiFileResponse> downloadFiles(@PathVariable String path) {
try {
File dir = new File(path);
if (!dir.exists() || !dir.isDirectory()) {
return ResponseEntity.notFound().build();
}
MultiFileResponse response = new MultiFileResponse();
for (File file : dir.listFiles()) {
if (file.isFile()) {
byte[] bytes = Files.readAllBytes(file.toPath());
response.addFile(file.getName(), bytes);
} else if (file.isDirectory()) {
// 如果是子目录,递归处理
response.addDirectory(file.getName(), downloadFiles(file.getAbsolutePath()));
}
}
return ResponseEntity.ok(response);
} catch (IOException e) {
throw new RuntimeException("Error reading files", e);
}
}
private static MultiFileResponse addDirectory(String name, MultiFileResponse parentResponse) {
// 这里可以继续将子目录的内容添加到parentResponse中
return parentResponse; // 省略了实际处理逻辑,仅作示例
}
class MultiFileResponse {
List<DownloadItem> files = new ArrayList<>();
void addFile(String fileName, byte[] content) {
files.add(new DownloadItem(fileName, content));
}
void addDirectory(String dirName, MultiFileResponse dirContent) {
files.add(new DownloadItem(dirName, null)); // 子目录暂存为null,实际可能需要创建链接或其他形式表示
}
// ...其他getters和setter忽略
}
class DownloadItem {
String name;
byte[] content;
// ...getter和setter忽略
}
}
```
在这个例子中,`/download/{path}` URL接收路径参数,然后检查是否存在并是目录。如果是文件,则读取其内容;如果是子目录,递归调用自身获取子目录的内容。返回结果封装在一个自定义的`MultiFileResponse`结构中。
阅读全文
相关推荐
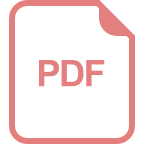
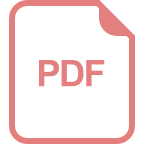
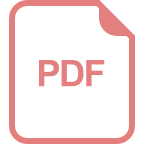
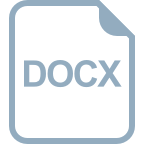
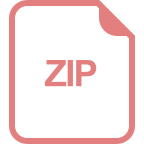
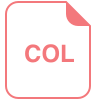












