写一个python代码要求实现从指定图片文件夹中随机选取指定数目的图片并将图片按顺序命名保存到指定文件夹,选取后要从原文件夹中删除随机选取的图片
时间: 2024-09-30 09:08:08 浏览: 34
你可以使用Python的os、shutil、random和PIL(Python Imaging Library)库来实现这个功能。下面是一个简单的示例代码:
```python
import os
import random
from PIL import Image
# 指定源图片文件夹路径
source_folder = '/path/to/source/folder'
# 目标图片保存文件夹路径
destination_folder = '/path/to/destination/folder'
# 需要选取的图片数量
num_of_images = 5
# 确保目标文件夹存在,如果不存在就创建
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# 获取源文件夹下的所有图片文件名列表
image_files = [img for img in os.listdir(source_folder) if img.endswith(('.jpg', '.png', '.jpeg'))]
# 如果图片数量小于需要选取的数量,打印提示并结束程序
if len(image_files) < num_of_images:
print(f"源文件夹内的图片不足{num_of_images}张,无法继续.")
else:
# 随机选择指定数量的图片文件名
selected_images = random.sample(image_files, num_of_images)
# 按顺序对选定的图片进行处理和保存
for i, image_name in enumerate(selected_images):
with Image.open(os.path.join(source_folder, image_name)) as img:
new_name = f'image_{i+1}.jpg' # 或者其他你想要的命名格式
img.save(os.path.join(destination_folder, new_name))
# 删除已选取的图片
os.remove(os.path.join(source_folder, image_name))
print("图片已经随机选取并保存完成.")
阅读全文
相关推荐
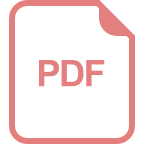
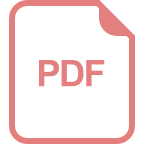
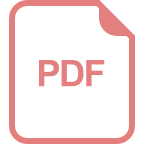
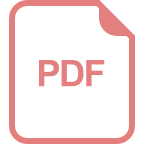
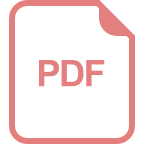
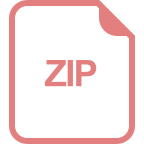
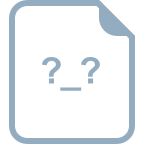
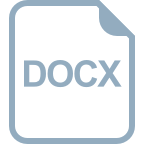
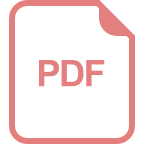
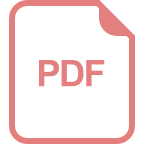
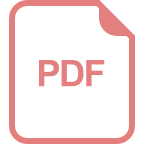
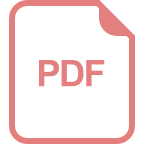
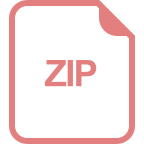
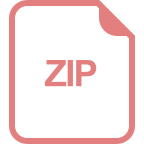
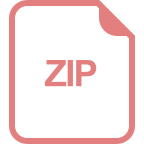
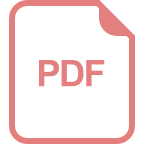
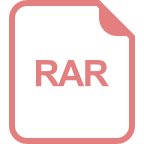