C语言用尾插法生成单链表
时间: 2024-10-06 12:02:50 浏览: 42
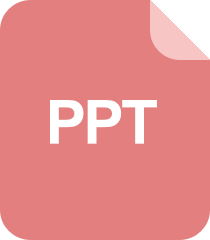
尾插法建单链表-C数据结构课件(线性表)
在C语言中,尾插法是一种高效地向单链表添加元素的方法。它的基本思想是在新节点的`next`指针指向当前链表的最后一个节点,而不是链表头部。以下是使用尾插法创建单链表的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并插入尾部
Node* tailInsert(Node* head, int data) {
// 如果链表为空,直接创建新节点作为头节点
if (head == NULL) {
head = (Node*)malloc(sizeof(Node));
head->data = data;
head->next = NULL;
} else {
// 否则遍历到链表尾部
Node* current = head;
while (current->next != NULL) {
current = current->next;
}
// 在尾部插入新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL; // 新节点的下一个节点为NULL,表示它已经是尾部
current->next = newNode;
}
return head;
}
int main() {
// 初始化链表
Node* list = NULL;
// 添加几个节点示例
list = tailInsert(list, 1);
list = tailInsert(list, 2);
list = tailInsert(list, 3);
// 打印链表内容
Node* temp = list;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
return 0;
}
```
在这个例子中,尾插法操作避免了每次添加新节点都要调整整个链表头部的复杂性,提高了插入操作的性能。
阅读全文
相关推荐
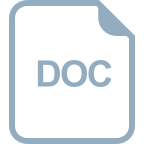
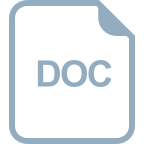

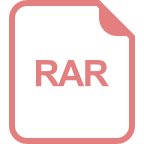
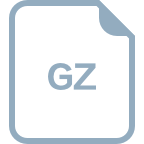
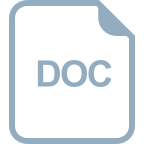


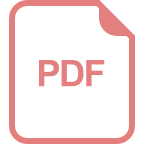
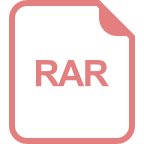
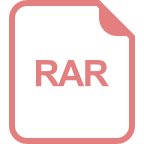
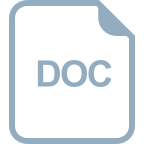
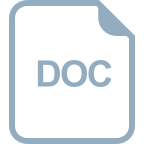
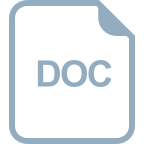
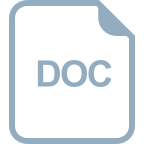
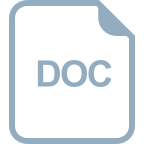
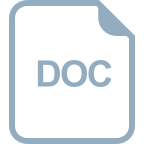