yolov5颜色识别
时间: 2023-10-10 10:14:47 浏览: 239
Yolov5是一个流行的目标检测算法,可以用于识别图像或视频中的各种不同对象。虽然Yolov5主要用于对象检测,但也可以通过对检测框中的像素进行处理来实现颜色识别。
要在Yolov5中实现颜色识别,可以按照以下步骤进行:
1. 准备数据集:收集包含不同颜色的图像数据集,并对每个颜色的对象进行标注。
2. 训练模型:使用Yolov5训练你的自定义数据集,确保标签中包含了颜色信息。
3. 推理和后处理:使用训练好的模型进行推理,获得检测框的位置和类别。然后,可以通过提取检测框内的像素并进行颜色分析来实现颜色识别。可以使用一些图像处理技术,如聚类、颜色直方图等来判断每个检测框中的主要颜色。
请注意,Yolov5本身并没有直接支持颜色识别的功能,需要进行一些额外的处理才能实现。这只是一种思路,具体的实现方法还需要根据你的具体需求和数据集进行适配。
相关问题
yolov5颜色识别原理
Yolov5是一种目标检测算法,它可以识别图像中的物体并标注出其边界框。至于颜色识别,实际上并不是yolov5的主要功能,但可以通过对训练数据的处理来实现。具体实现方法可以是在训练数据集中对颜色进行标注,然后在yolov5的模型中添加一个额外的输出通道来预测颜色信息。当然,这需要对yolov5的模型进行改进和重新训练,才能使其具备颜色识别的能力。
yolov5颜色识别的代码
以下是使用 YOLOv5 进行颜色识别的 Python 代码示例:
```python
import torch
import cv2
import numpy as np
from pathlib import Path
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.datasets import letterbox
# 加载模型
weights = 'yolov5s.pt'
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max())
names = model.module.names if hasattr(model, 'module') else model.names
# 颜色映射
colors = [
[255, 0, 0], # 红色
[0, 255, 0], # 绿色
[0, 0, 255], # 蓝色
[255, 255, 0], # 青色
[255, 0, 255], # 紫色
[0, 255, 255] # 黄色
]
# 预处理图像
def preprocess(img, size):
img = letterbox(img, new_shape=size)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).to(device)
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
return img
# 检测颜色
def detect_color(img, conf_threshold=0.5, iou_threshold=0.4):
img_size = img.shape[:2]
img_preprocessed = preprocess(img, size=model.img_size)
detections = model(img_preprocessed)[0]
detections = non_max_suppression(detections, conf_threshold, iou_threshold, classes=None, agnostic=True)
color_labels = []
for detection in detections:
if detection is not None and len(detection):
detection = scale_coords(img_size, detection[:, :4], img_preprocessed.shape[2:]).round()
for *xyxy, conf, cls in reversed(detection):
color_label = names[int(cls)]
color_labels.append(color_label)
return color_labels
# 加载图像
img_path = 'example.jpg'
img = cv2.imread(img_path)
# 检测颜色
color_labels = detect_color(img)
# 绘制检测结果
for i, color_label in enumerate(color_labels):
xy = (10, 30 * i + 30)
color = colors[i % len(colors)]
cv2.putText(img, color_label, xy, cv2.FONT_HERSHEY_SIMPLEX, 1, color, 2)
cv2.rectangle(img, (10, 30 * i + 10), (200, 30 * i + 40), color, -1)
# 显示结果
cv2.imshow('Color Detection', img)
cv2.waitKey()
cv2.destroyAllWindows()
```
请注意,此示例代码假定您已经安装了 YOLOv5,并且已经下载了预训练权重文件 `yolov5s.pt`。如果您还没有安装和下载,可以按照以下步骤进行操作:
1. 安装 YOLOv5:`pip install yolov5`
2. 下载预训练权重文件 `yolov5s.pt`:`wget https://github.com/ultralytics/yolov5/releases/download/v5.0/yolov5s.pt`
相关推荐
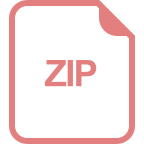
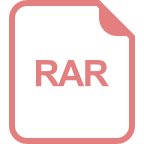
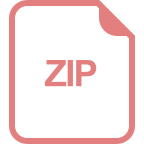












