okhttp3实现动态代理springboot
时间: 2024-06-26 14:01:25 浏览: 3
OkHttp3 是一个强大的 HTTP 客户端库,它提供了灵活的 API 和丰富的功能,包括支持网络连接池、断点续传、缓存控制等。要在 Spring Boot 中使用 OkHttp3 实现动态代理,通常是为了方便进行性能测试、日志跟踪或安全控制,例如使用像 Charles 或 WireShark 这样的代理服务器。
以下是一个简单的步骤来配置 OkHttp3 使用动态代理:
1. 添加依赖:首先,在你的 `build.gradle` 文件中添加 OkHttp3 的依赖(如果你还没有添加):
```groovy
implementation 'com.squareup.okhttp3:okhttp:4.9.3'
```
2. 配置代理:在 Spring Boot 的配置类(如 `ApplicationConfig` 或 `OkHttpClientConfig`)中设置 OkHttpClient 的代理:
```java
import okhttp3.OkHttpClient;
import okhttp3.Proxy;
import okhttp3.logging.HttpLoggingInterceptor;
@Configuration
public class OkHttpClientConfig {
@Value("${proxy.host:}")
private String proxyHost;
@Value("${proxy.port:}")
private int proxyPort;
@Bean
public OkHttpClient httpClient() {
OkHttpClient.Builder builder = new OkHttpClient.Builder();
if (StringUtils.isNotBlank(proxyHost) && proxyPort > 0) {
Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress(proxyHost, proxyPort));
builder.proxy(proxy);
HttpLoggingInterceptor loggingInterceptor = new HttpLoggingInterceptor();
loggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
builder.addInterceptor(loggingInterceptor);
}
// 其他配置,如连接池、超时设置等
return builder.build();
}
}
```
这里假设你已经有了一个环境变量 `proxy.host` 和 `proxy.port` 来定义代理服务器的地址和端口。如果没有,你需要根据实际情况提供这些值。
3. 注册为 RestTemplate 或其他依赖的默认客户端:如果你打算使用 OkHttp3 作为 Spring Web 的 `RestTemplate` 或其他客户端的默认实现,你可以将 OkHttpClient 注册到 `WebClient` 或 `RestTemplate` 中:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.client.OkHttp3ClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
@Autowired
public void configureRestTemplate(OkHttpClient httpClient) {
RestTemplate restTemplate = new RestTemplate(new OkHttp3ClientHttpRequestFactory(httpClient));
// 使用 restTemplate 发送 HTTP 请求
}
```
相关推荐
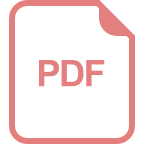
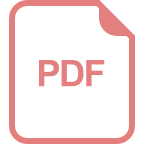
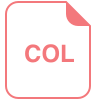
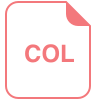
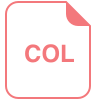
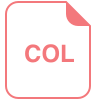
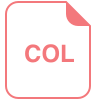









