android自定义控件ObjectAnimator翻转按钮示例代码
时间: 2024-12-20 08:15:55 浏览: 8
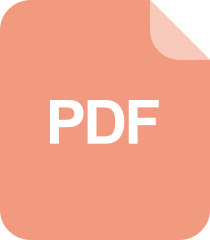
Android自定义控件之翻转按钮的示例代码
在Android中,你可以使用`ObjectAnimator`来自定义动画效果,例如创建一个翻转按钮的动画。首先,你需要在XML布局中声明一个普通的按钮,并设置一些基础属性,比如宽度、高度和背景颜色:
```xml
<Button
android:id="@+id/myButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@android:color/holo_blue_light"
android:text="Flip Me" />
```
然后,在Java或Kotlin代码中,你可以动态创建一个`ObjectAnimator`并设置动画属性来实现翻转动画:
**Java示例**:
```java
import android.animation.ObjectAnimator;
import android.view.View;
// ...
public void flipButton(View button) {
// 获取当前按钮的位置信息
float centerX = button.getX() + button.getWidth() / 2;
float centerY = button.getY() + button.getHeight() / 2;
// 创建一个旋转动画
ObjectAnimator rotateAnim = ObjectAnimator.ofFloat(button, "rotation", 0f, 180f);
rotateAnim.setDuration(500); // 设置动画持续时间
// 添加动画完成后的回调,这里可以处理翻转后的状态
rotateAnim.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
button.setTranslationX(centerY);
button.setTranslationY(-centerX);
button.setRotation((float) animation.getAnimatedValue());
}
});
// 开始动画
rotateAnim.start();
}
```
**Kotlin示例**:
```kotlin
import android.animation.ObjectAnimator
import android.view.View
// ...
fun flipButton(button: View) {
val centerX = button.x + button.width.toFloat() / 2
val centerY = button.y + button.height.toFloat() / 2
val rotateAnim = ObjectAnimator.ofFloat(button, "rotation", 0f, 180f)
rotateAnim.duration = 500L // 设置动画持续时间
// 更新监听器
rotateAnim.addUpdateListener { animation ->
button.translationX = centerY
button.translationY = -centerX
button.rotation = animation.animatedValue as Float
}
// 开始动画
rotateAnim.start()
}
```
在这个例子中,动画会将按钮绕中心点顺时针旋转180度,达到翻转的效果。
阅读全文
相关推荐
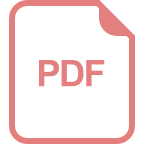
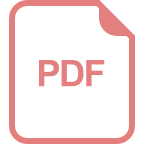
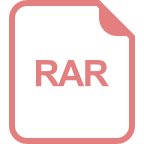
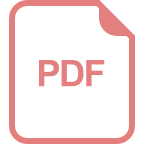
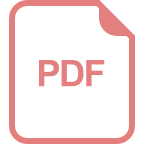
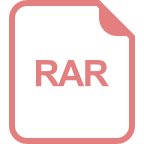
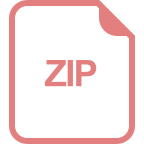
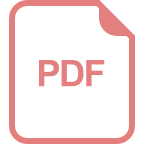
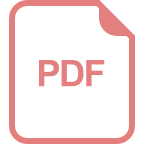
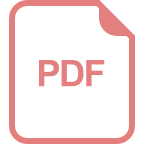
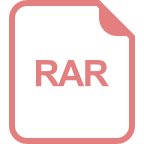
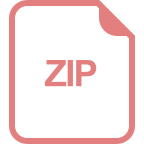
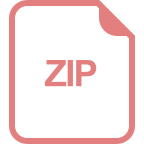
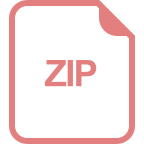