C#调用ftps和ftp的区别
时间: 2024-10-12 17:02:32 浏览: 42
在C#中,FTPS (File Transfer Protocol over SSL/TLS) 和 FTP (Plain File Transfer Protocol) 都用于文件传输,但它们之间有显著的安全性和加密方面的区别:
1. **FTP** (基础版):
- 使用明文连接,这意味着数据在传输过程中不被加密,可能存在信息泄露风险。
- 协议版本通常为FTP v1.0或v1.1。
2. **FTPS** (安全版):
- 基于SSL/TLS协议对数据进行加密,提供更高级别的安全保护,防止中间人攻击和数据窥探。
- 支持FTP SFTP (SSH File Transfer Protocol) 和 FTPES (FTP with Explicit Security),分别基于TCP和TLS。
- 协议版本通常更高,如FTP v1.97、FTP v2等,并且支持FTP Over TLS (FTP with Control Channel Encryption).
在C#中调用这两种服务,你需要使用相应的库。对于FTP,你可以使用`System.Net.FtpClient`类;对于FTPS,可以使用`FtpSClient`或`FtpWebRequest`(如果使用的是FTPES)。需要注意的是,FTPS需要服务器支持并配置SSL/TLS,而FTP则不需要。
相关问题
C#调用ftps方式
在C#中,你可以使用FTPClient类或者第三方库如FluentFTP来通过ftps (FTP over SSL)方式连接到FTP服务器。以下是基本步骤:
1. 引入所需命名空间:
```csharp
using System.Net;
using System.IO;
```
2. 创建FTPClient实例并启用SSL/TLS:
```csharp
FtpWebRequest request = (FtpWebRequest)WebRequest.Create("ftp://[username]:[password]@[server地址]/");
request.Method = WebRequestMethods.Ftp.ListDirectory;
request.UseSsl = true; // 如果是ftps,则设为true
```
3. 设置认证和代理(如果需要):
```csharp
request.Credentials = new NetworkCredential("[用户名]", "[密码]");
if (!string.IsNullOrEmpty(proxyAddress))
request.Proxy = new WebProxy(proxyAddress);
```
4. 执行请求并处理响应:
```csharp
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
StreamReader reader = new StreamReader(response.GetResponseStream());
string directoryListing = reader.ReadToEnd();
reader.Close();
response.Close();
```
5. 关闭连接:
```csharp
((IDisposable)request).Dispose();
```
C#调用ftps接口
在C#中,你可以使用`System.Net.FtpsClient`类来调用FTP over SSL (ftps)接口。这是一个用于安全文件传输的FTP客户端库,让你能够通过SSL/TLS加密连接到FTP服务器。
以下是一个简单的示例,展示如何创建一个FtpsClient实例并执行基本操作:
```csharp
using System;
using System.IO;
using System.Net;
class Program
{
static void Main()
{
try
{
// 创建FtpsClient实例
FtpsClient ftpsClient = new FtpsClient("ftpssl://yourserver.com");
// 登录(如果需要)
ftpsClient.Credentials = new NetworkCredential("username", "password");
// 进入目录
ftpsClient.ChangeDirectory("/path/to/directory");
// 上传文件
using (FileStream sourceStream = File.OpenRead(@"本地文件路径\file.txt"))
{
ftpsClient.UploadFile("remote_file_path.txt", sourceStream);
}
// 下载文件
string remoteFilePath = "remote_file_path.txt";
using (FileStream destinationStream = File.Create(@"本地保存路径\downloaded_file.txt"))
{
ftpsClient.DownloadFile(remoteFilePath, destinationStream);
}
// 关闭连接
ftpsClient.Disconnect();
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
阅读全文
相关推荐





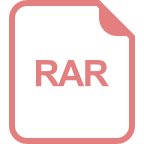






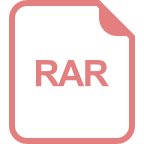
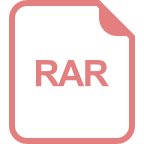

