vue上传图片的组件
时间: 2024-09-06 08:01:56 浏览: 39
Vue.js 中上传图片通常会使用内置的 `vue-file-upload` 或者 `element-ui`、`iview` 等前端 UI 框架提供的文件上传组件。这里简单介绍一种基本的方法:
**使用 Vue-File-Upload 组件**:
首先,你需要安装这个插件,通过 npm 安装:
```bash
npm install vue-file-upload axios
```
然后在组件中导入并使用它:
```html
<template>
<div>
<input type="file" @change="handleImageChange" />
<button @click="uploadImage">上传</button>
<p v-if="imageUrl">{{ imageUrl }}</p> <!-- 显示已选图片预览 -->
</div>
</template>
<script>
import Vue from 'vue';
import FileUploader from 'vue-file-upload';
export default {
components: {
FileUploader,
},
data() {
return {
imageUrl: '',
};
},
methods: {
handleImageChange(e) {
this.$refs.uploader.addFiles(e.target.files);
},
uploadImage() {
this.$refs.uploader.submit({
url: '/api/upload', // 替换为你的实际上传API地址
method: 'POST',
onProgress: (progressEvent) => {
console.log(`上传进度: ${Math.round(progressEvent.loaded * 100 / progressEvent.total)}%`);
},
onSuccess(response) {
if (response.data && response.data.url) {
this.imageUrl = response.data.url;
}
},
onError(error) {
console.error('上传错误:', error);
},
});
},
},
};
</script>
```
在这个例子中,用户选择文件后,会触发 `handleImageChange` 方法添加到上传队列,点击“上传”按钮则会发起请求。
**使用 Element-UI 的 el-upload 组件**:
Element UI 提供了更丰富的表单组件,你可以这样使用:
```html
<template>
<el-upload
ref="upload"
:action="uploadUrl"
:on-change="handleChange"
:before-upload="beforeUpload"
:on-success="handleSuccess"
:on-error="handleError"
>
<i class="el-icon-plus"></i>
<div slot="tip">点击上传图片</div>
</el-upload>
<img :src="imageUrl" v-if="imageUrl" alt="图片预览">
</template>
<script>
import { ref } from 'vue';
import { ElUpload } from 'element-plus';
export default {
components: {
ElUpload,
},
setup(props) {
const uploadUrl = props.uploadUrl || '/api/upload'; // 设置上传URL
const imageUrl = ref('');
const handleChange = ({ file }) => {
this.imageUrl = URL.createObjectURL(file);
};
const beforeUpload = (file) => {
// 这里可以做些验证或者处理,例如限制文件类型、大小等
};
const handleSuccess = (res, file) => {
if (res.data && res.data.url) {
this.imageUrl = res.data.url;
}
};
const handleError = (error, file) => {
console.error('上传失败:', error);
};
return { imageUrl, uploadUrl, handleChange, beforeUpload, handleSuccess, handleError };
},
};
</script>
```
以上就是使用 Vue 上传图片的基本组件示例,具体的样式和配置可能会根据实际需求有所不同。
阅读全文
相关推荐
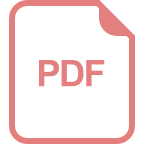
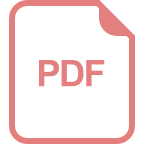
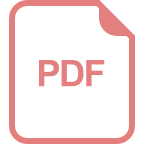
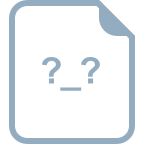
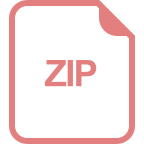
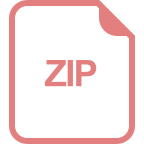
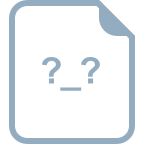
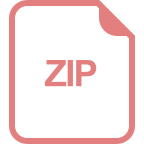
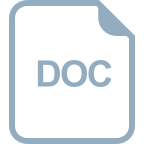
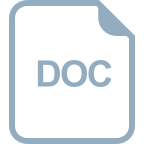
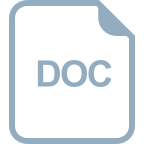
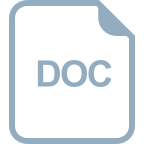


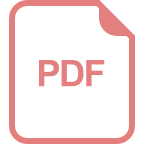
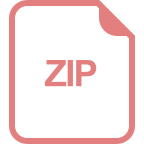
