pdf是用excel生成然后转为pdf
时间: 2023-11-09 16:53:35 浏览: 41
将Excel文件转换为PDF文件的方法有多种。以下是一种常见的方法,使用Apache POI库读取Excel文件并使用iText库生成PDF文件:
1. 添加依赖项:在`pom.xml`文件中添加以下依赖项,以引入Apache POI和iText库:
```xml
<dependencies>
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<!-- iText -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
</dependencies>
```
2. 读取Excel文件并生成PDF文件:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.*;
public class ExcelToPdfConverter {
public static void main(String[] args) {
String excelFilePath = "path/to/excel/file.xlsx";
String pdfFilePath = "output.pdf";
try {
// 读取Excel文件
FileInputStream fis = new FileInputStream(new File(excelFilePath));
Workbook workbook = new XSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
// 创建PDF文件
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream(pdfFilePath));
document.open();
// 遍历Excel中的每一行并将内容写入PDF
for (Row row : sheet) {
for (Cell cell : row) {
String cellValue = "";
if (cell.getCellType() == CellType.STRING) {
cellValue = cell.getStringCellValue();
} else if (cell.getCellType() == CellType.NUMERIC) {
cellValue = String.valueOf(cell.getNumericCellValue());
}
document.add(new com.itextpdf.text.Paragraph(cellValue));
}
}
// 关闭文档和文件流
document.close();
workbook.close();
fis.close();
System.out.println("PDF文件生成成功:" + pdfFilePath);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述示例代码中,将`excelFilePath`替换为实际的Excel文件路径,并将`pdfFilePath`替换为希望生成的PDF文件路径。然后运行该代码,它将读取Excel文件的内容,并将内容写入到PDF文件中。
请注意,上述代码以逐行逐列的方式将Excel数据写入PDF文件,你可能需要根据实际需求进行调整和优化。另外,确保在使用完文件流后关闭它们,以释放资源。
相关推荐
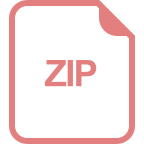
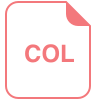













