c++如何获取绝对值
时间: 2024-09-22 11:04:05 浏览: 64
在C++中,获取一个数的绝对值可以使用内置的库函数`abs()`,它是`cstdlib`头文件的一部分。这个函数接受一个整型或长整型数值作为输入,并返回其对应的非负值。例如:
```cpp
#include <cstdlib>
int main() {
int num = -5;
int absoluteValue = abs(num); // 输出:5
long long negativeLong = -999999999LL;
long long absoluteLong = abs(negativeLong); // 输出:999999999LL
return 0;
}
```
如果你需要处理浮点数,也可以使用`<cmath>`头文件中的`fabs()`函数,它同样接收一个double或float类型的参数:
```cpp
#include <cmath>
double floatingNum = -3.14;
double absoluteFloating = fabs(floatingNum); // 输出:3.14
return 0;
```
相关问题
c++ 求绝对值
C++ 中求绝对值可以使用标准库中的 abs 函数。例如:
```c++
#include <cstdlib> // 包含 abs 函数的头文件
int main() {
int x = -10;
int abs_x = abs(x); // 求 x 的绝对值
return 0;
}
```
如果要求浮点数的绝对值,可以使用标准库中的 fabs 函数。例如:
```c++
#include <cmath> // 包含 fabs 函数的头文件
int main() {
double x = -1.23;
double abs_x = fabs(x); // 求 x 的绝对值
return 0;
}
```
注意,求绝对值的函数的参数类型必须是整型或浮点型。
QT C++求绝对值
在QT C++中,可以使用abs()函数来求绝对值。该函数的原型如下:
```cpp
int abs(int n);
```
其中,n为要求绝对值的整数。该函数会返回n的绝对值。
以下是一个示例代码:
```cpp
#include <iostream>
#include <cstdlib>
int main() {
int n = -5;
int absValue = abs(n);
std::cout << "The absolute value of " << n << " is " << absValue << std::endl;
return 0;
}
```
输出结果为:
```
The absolute value of -5 is 5
```
阅读全文
相关推荐
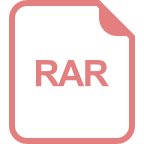
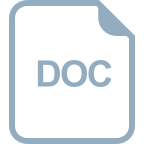












