C++queue find()函数
时间: 2024-10-03 21:04:34 浏览: 48
C++中的`std::queue`是一个容器适配器,它实现了双端队列(deque)的后进先出(LIFO,Last In First Out)特性。`find()`函数并非`std::queue`的标准成员函数,这个函数通常存在于`std::vector`、`std::list`等容器中,用于在一个范围查找特定元素。
然而,在`std::queue`中,如果你想搜索队列中的元素,你可以通过迭代器来进行遍历。例如:
```cpp
template <typename T>
class queue {
public:
// ... 其他成员函数
bool contains(const T& value) {
std::queue<T>::iterator it = front();
while (it != end()) {
if (*it == value) {
return true;
}
++it;
}
return false;
}
};
```
上述代码中,`contains`函数会检查队列中的每个元素是否等于给定的值`value`。如果找到,则返回`true`,否则返回`false`。
相关问题
c++ queue 的find
C++中的std::queue是一个容器适配器,它提供了先进先出(FIFO)的数据结构。由于std::queue是一种封装好的数据结构,它没有提供直接的find操作。但是,你可以通过以下方法来实现查找:
1. 将队列转换为其他容器类型:你可以将std::queue转换为std::vector或std::deque等支持查找操作的容器类型,然后使用这些容器类型的find函数进行查找。
```cpp
std::queue<int> myQueue;
// 添加一些元素到队列myQueue
std::vector<int> vec(myQueue.c.begin(), myQueue.c.end());
auto it = std::find(vec.begin(), vec.end(), target);
if (it != vec.end()) {
// 找到了目标元素
}
```
2. 遍历队列进行查找:你可以遍历队列,一个一个元素地进行比较,直到找到目标元素或者遍历结束。
```cpp
std::queue<int> myQueue;
// 添加一些元素到队列myQueue
bool found = false;
while (!myQueue.empty()) {
int element = myQueue.front();
myQueue.pop();
if (element == target) {
// 找到了目标元素
found = true;
break;
}
}
if (found) {
// 找到了目标元素
}
```
请注意,以上方法都需要遍历队列,并且时间复杂度为O(n),其中n是队列中的元素个数。如果你需要经常进行查找操作,可能需要考虑使用其他数据结构来满足你的需求。
c++中的queue如何使用find函数
在C++中,queue是一个标准模板库(STL)容器,是一种先进先出的数据结构。queue不支持查找操作,因为它没有提供迭代器访问元素。如果需要查找元素,可以将queue转换为其他支持查找操作的容器,如vector或list。
以下是将queue转换为vector并使用find函数查找元素的示例代码:
```c++
#include <iostream>
#include <queue>
#include <vector>
#include <algorithm>
int main()
{
std::queue<int> q;
q.push(10);
q.push(20);
q.push(30);
// Convert queue to vector
std::vector<int> v(q.cbegin(), q.cend());
// Find element in vector
auto it = std::find(v.cbegin(), v.cend(), 20);
if (it != v.cend())
{
std::cout << "Element found at position: " << it - v.cbegin() << std::endl;
}
else
{
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
输出:
```
Element found at position: 1
```
阅读全文
相关推荐
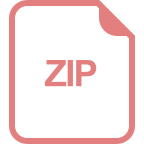
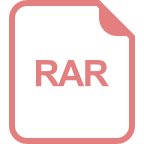
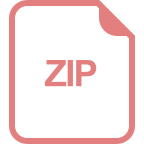
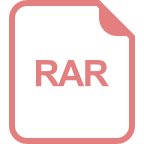
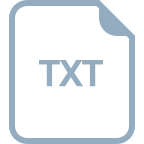
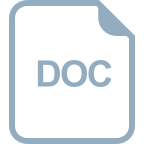
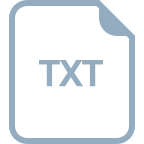
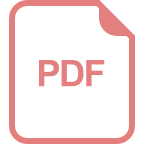
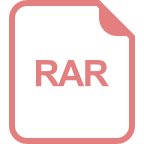
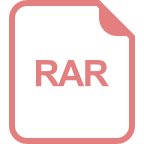
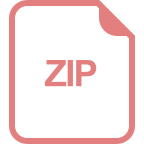
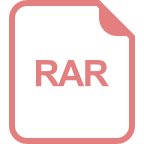
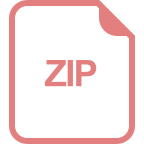
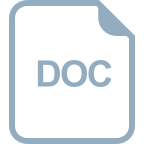
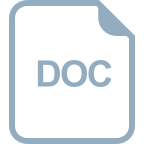
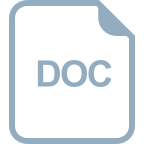
