java实现Apache Commons Codec将图片转为Base64编码的逻辑
时间: 2024-04-16 16:27:06 浏览: 19
要使用Apache Commons Codec将图片转为Base64编码,你可以按照以下步骤进行操作:
1. 首先,确保你已经将Apache Commons Codec库添加到你的项目中。你可以在Maven中添加以下依赖项:
```xml
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.15</version>
</dependency>
```
2. 然后,在你的Java代码中,导入相关的类:
```java
import org.apache.commons.codec.binary.Base64;
```
3. 在`getImageBase64`方法中,实现将图片转为Base64编码的逻辑。以下是一个示例代码:
```java
import org.apache.commons.codec.binary.Base64;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class BaiduOCR {
// ...
// 将图片转为Base64编码
private static String getImageBase64(String imagePath) throws IOException {
File file = new File(imagePath);
FileInputStream inputStream = new FileInputStream(file);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
byte[] imageBytes = outputStream.toByteArray();
String base64String = Base64.encodeBase64String(imageBytes);
inputStream.close();
outputStream.close();
return base64String;
}
// ...
}
```
在上述代码中,我们首先打开图片文件,然后使用`FileInputStream`读取文件内容,并使用`ByteArrayOutputStream`将文件内容写入一个字节数组缓冲区。之后,我们调用`Base64.encodeBase64String`方法将字节数组转为Base64编码的字符串。
请注意替换`imagePath`变量为你实际的图片路径。
这样,你就可以使用Apache Commons Codec将图片转为Base64编码了。如果有任何其他问题,请随时提问。
相关推荐
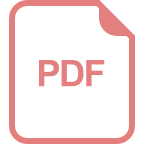
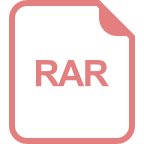
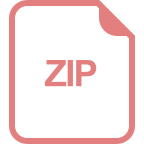














