react 父组件调用字方法
时间: 2023-11-03 21:18:00 浏览: 157
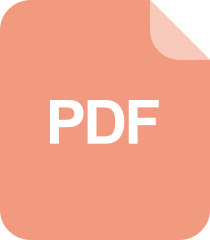
react 兄弟组件如何调用对方的方法示例

在 React 中,父组件调用子组件的方法有两种方式:
1. 使用 Refs:
在父组件中,通过创建一个引用(ref)并将其传递给子组件,然后可以使用这个引用来调用子组件的方法。下面是一个示例:
```jsx
class ParentComponent extends React.Component {
constructor(props) {
super(props);
this.childRef = React.createRef();
}
handleClick = () => {
this.childRef.current.childMethod();
}
render() {
return (
<div>
<ChildComponent ref={this.childRef} />
<button onClick={this.handleClick}>调用子组件方法</button>
</div>
);
}
}
class ChildComponent extends React.Component {
childMethod() {
console.log("子组件方法被调用");
}
render() {
return (
<div>子组件</div>
);
}
}
```
在上面的示例中,通过 `React.createRef()` 创建一个引用 `childRef`,并将其传递给 `ChildComponent`,然后在父组件的 `handleClick` 方法中可以使用 `childRef.current` 来访问子组件的实例,并调用子组件的方法。
2. 通过 Props:
父组件可以通过将一个函数作为 props 传递给子组件,在子组件内部调用该函数来实现与父组件的通信。下面是一个示例:
```jsx
class ParentComponent extends React.Component {
handleChildMethod = () => {
console.log("子组件方法被调用");
}
render() {
return (
<div>
<ChildComponent handleMethod={this.handleChildMethod} />
</div>
);
}
}
class ChildComponent extends React.Component {
render() {
return (
<div>
子组件
<button onClick={this.props.handleMethod}>调用父组件方法</button>
</div>
);
}
}
```
在上面的示例中,通过将 `handleChildMethod` 函数作为 `handleMethod` props 传递给子组件 `ChildComponent`,然后在子组件内部可以通过 `this.props.handleMethod()` 调用父组件的方法。
这两种方式都可以让父组件调用子组件的方法,具体使用哪种方式取决于你的需求和项目的架构。
阅读全文
相关推荐
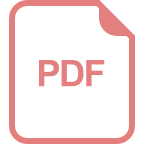
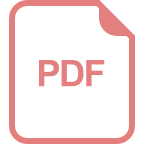
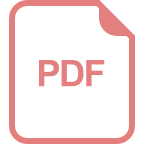
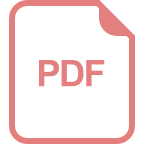
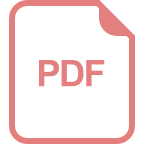
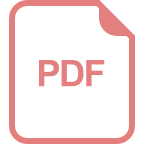
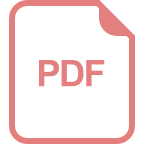
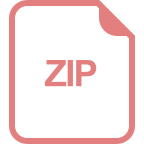
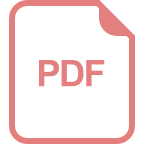
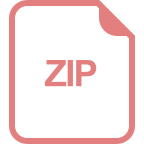
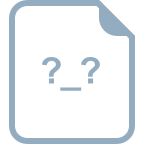
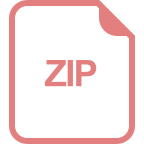
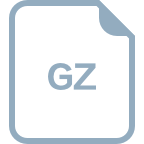
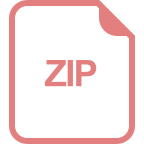
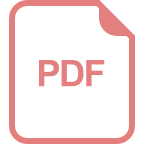
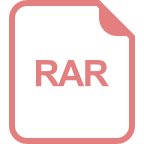