list集合存user对象,对象有个date属性,使用stream查出最大时间
时间: 2024-10-23 21:10:05 浏览: 31
当你有一个List<User>类型的集合,其中User对象包含一个date属性,你可以使用Java Stream API来找出其中日期最大的用户。首先,你需要导入Stream相关的包,然后可以按照以下步骤操作:
```java
import java.time.LocalDate;
import java.util.List;
import java.util.stream.Collectors;
public LocalDate findMaxDate(List<User> userList) {
return userList.stream()
.map(User::getDate) // 获取每个User对象的date属性
.max(Comparator当地类比自然排序) // 使用LocalDate的自然顺序比较
.orElse(null); // 如果列表为空,则返回null
}
// 后续如果需要获取对应的User对象,可以在findMaxDate方法内部处理
Optional<User> maxDateUser = userList.stream()
.filter(user -> user.getDate().equals(findMaxDate(userList)))
.findFirst();
相关问题
在springboot项目中使用queryWrapper判断对象属性是否为空,属性类型包含String,int,Date
在Spring Boot项目中,你可以使用QueryWrapper类来构建查询条件,并根据对象的属性进行判断。下面是一些示例代码,演示如何使用QueryWrapper来判断对象属性是否为空,并处理String、int和Date类型的属性。
首先,确保你已经导入了Spring Data JPA依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
接下来,假设你有一个实体类,例如User,包含String类型的属性name、int类型的属性age和Date类型的属性birthday。你可以根据对象的属性类型进行查询条件判断,代码如下:
```java
import org.springframework.data.jpa.domain.Specification;
import org.springframework.data.util.Pair;
import org.springframework.stereotype.Component;
import java.util.List;
import java.util.stream.Collectors;
@Component
public class UserQueryService {
public List<User> getUsersWithNullProperties() {
// 构建查询条件,判断属性是否为空
Specification<User> specification = (root, query, builder) -> {
// 判断name是否为空
if (root.get("name") == null) {
return true;
}
// 判断age是否为空
if (root.get("age") == null) {
return true;
}
// 判断birthday是否为空
if (root.get("birthday") == null) {
return true;
}
return false; // 如果所有属性都不为空,则返回false,表示不满足查询条件
};
// 执行查询并返回结果
return userRepository.findAll(specification).stream()
.map(User::getUsername) // 根据实际需要获取需要的属性值,这里假设获取username属性值
.collect(Collectors.toList());
}
}
```
上述代码中,我们使用了QueryWrapper的Specification接口来构建查询条件。通过root对象获取属性的值,判断是否为空。如果所有属性都不为空,则返回false,表示不满足查询条件。根据实际需要,你可以根据不同的属性类型进行不同的判断和处理。
请注意,上述代码只是一个简单的示例,实际应用中可能需要根据具体需求进行适当的修改和扩展。另外,确保你已经配置了相应的数据源和Repository接口。
@RequestMapping("addMsgList.action") public String addMsgList( String businessid,String msg){ this.front(); if (this.getSession().getAttribute("userid") == null) { return "redirect:/index/preLogin.action"; } String userid = (String) this.getSession().getAttribute("userid"); MyMsgList list=new MyMsgList(); list.setUserid(userid); list.setBusinessid(businessid); List<MyMsgList> lists = msgService.listByUser(userid); if (lists.size()>0){ List<String> collect = lists.stream().map(t -> t.getBusinessid()).collect(Collectors.toList()); if (!collect.contains(businessid)){ //添加链接 msgService.addMsgList(list); } }else { //添加链接 msgService.addMsgList(list); } MyMsgs obj=new MyMsgs(); obj.setMsg(msg); obj.setBusinessid(businessid); obj.setUsersid(userid); return addMsg(obj); } /** * 新增留言 * @return */ @RequestMapping("addMsg.action") public String addMsg(MyMsgs myMsgs){ Date date = new Date(); DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //创建日期的对象,通过format()方法将日期对象格式化,格式化后是String类型 String s = dateFormat.format(date); myMsgs.setCreatetime(s); myMsgs.setMsgtype("1"); myMsgs.setMid(s); msgService.addMsg(myMsgs); return "redirect:/index/myMsg.action?bid="+myMsgs.getBusinessid(); }的关键代码是哪部分
关键代码如下:
```
@RequestMapping("addMsgList.action")
public String addMsgList(String businessid, String msg) {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
String userid = (String) this.getSession().getAttribute("userid");
MyMsgList list = new MyMsgList();
list.setUserid(userid);
list.setBusinessid(businessid);
List<MyMsgList> lists = msgService.listByUser(userid);
if (lists.size() > 0) {
List<String> collect = lists.stream().map(t -> t.getBusinessid()).collect(Collectors.toList());
if (!collect.contains(businessid)) {
//添加链接
msgService.addMsgList(list);
}
} else {
//添加链接
msgService.addMsgList(list);
}
MyMsgs obj = new MyMsgs();
obj.setMsg(msg);
obj.setBusinessid(businessid);
obj.setUsersid(userid);
return addMsg(obj);
}
@RequestMapping("addMsg.action")
public String addMsg(MyMsgs myMsgs) {
Date date = new Date();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//创建日期的对象,通过format()方法将日期对象格式化,格式化后是String类型
String s = dateFormat.format(date);
myMsgs.setCreatetime(s);
myMsgs.setMsgtype("1");
myMsgs.setMid(s);
msgService.addMsg(myMsgs);
return "redirect:/index/myMsg.action?bid=" + myMsgs.getBusinessid();
}
```
其中,`addMsgList` 方法用于添加留言列表,`addMsg` 方法用于添加留言。在 `addMsgList` 方法中,首先获取当前用户的 `userid`,然后创建一个 `MyMsgList` 对象,并设置其 `userid` 和 `businessid` 属性。接着通过 `msgService` 对象调用 `listByUser` 方法获取当前用户的留言列表,如果留言列表中已经存在该 `businessid`,则不进行操作,否则将该 `MyMsgList` 对象添加到数据库中。最后,创建一个 `MyMsgs` 对象,设置其各个属性,并调用 `addMsg` 方法将该留言添加到数据库中,并跳转到 `myMsg.action` 页面。
阅读全文
相关推荐
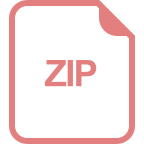
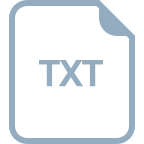
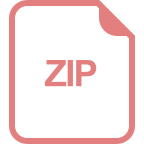
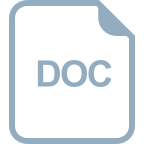
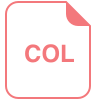
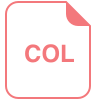
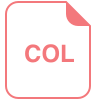
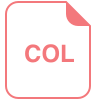
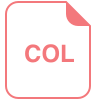
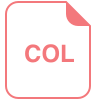
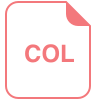
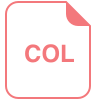
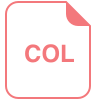



