c语言编程实现BF模式匹配算法,要求如下: 1. 从键盘输入主串和模式串; 2. 匹配成功时输出模式串在主串中第一次出现的位序,匹配不成功时显示相应的提示信息。
时间: 2024-11-24 10:40:01 浏览: 42
BF(Boyer-Moore)模式匹配算法是一种用于快速查找字符串中特定模式的高效算法。在C语言中实现BF算法,首先需要了解基本步骤:
1. **预处理阶段**:
- 计算模式串的后缀数组,存储每个字符最后一次出现的位置。
- 计算坏字符表(Bad Character Heuristic),记录模式串中每个字符到其最右边的相同字符的距离。
2. **搜索阶段**:
- 输入主串和模式串。
- 设置两个指针,`i`遍历主串,`j`遍历模式串。
- 当`j`指向模式串结束时,说明找到一次匹配;否则,检查以下条件:
a. 如果当前字符不匹配,先尝试后缀匹配(如果`m > i - j + pattern[j]`)。
b. 否则,尝试坏字符表匹配(`pattern[j] != main[i - j]`时移动`main`指针)。
以下是简单的C语言代码实现BF模式匹配算法:
```c
#include <stdio.h>
#include <string.h>
void preprocess(char* pattern, int length) {
// ...后缀数组和坏字符表计算...
}
int boyer_moore_search(char* main_str, char* pattern, int m) {
int i = 0, j = 0;
preprocess(pattern, strlen(pattern));
while (i <= main_str_len - m) {
if (main_str[i] == pattern[j]) {
if (j == m - 1) { // match found
printf("Pattern found at position %d\n", i - j);
return i;
}
else {
i++;
j++;
}
} else {
int skip = 0;
if (j > 0 && pattern[j] != pattern[j - 1]) {
skip = max(skip, pattern[j] - main_str[i - j]);
} else {
skip = max(skip, bad_char_table[pattern[j]]);
}
i += skip;
}
}
printf("Pattern not found in the main string.\n");
return -1;
}
int main() {
char main_str[100], pattern[100];
printf("Enter the main string: ");
fgets(main_str, sizeof(main_str), stdin);
main_str[strcspn(main_str, "\n")] = '\0';
printf("Enter the pattern string: ");
fgets(pattern, sizeof(pattern), stdin);
pattern[strcspn(pattern, "\n")] = '\0';
int main_str_len = strlen(main_str);
int result = boyer_moore_search(main_str, pattern, strlen(pattern));
return 0;
}
```
阅读全文
相关推荐




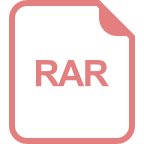





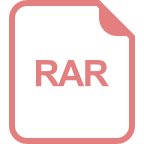


