matlab走迷宫最短路径
时间: 2024-06-22 12:03:21 浏览: 8
在MATLAB中,解决迷宫中的最短路径问题通常可以使用图论的方法,比如广度优先搜索(Breadth-First Search, BFS)或Dijkstra算法。这两个算法常用于寻找两点之间的最短路径。下面是使用BFS的一个基本示例:
**使用BFS解决迷宫最短路径:**
1. **创建迷宫矩阵**:首先,你需要将迷宫表示为一个二维数组,0代表可以通行,1代表墙壁。
2. **初始化**:设置起点和终点,定义一个队列来存储节点,并标记起点为已访问。
3. **广度优先遍历**:
a. 将起点加入队列。
b. 当队列不为空时,从队首取出一个节点,如果它是终点,则找到路径;如果不是,检查其邻居(上下左右)是否可通行且未访问过,可通行则标记为已访问并加入队列。
c. 重复步骤b,直到队列为空或找到终点。
4. **路径记录**:在找到路径后,可以通过回溯记录下每个节点,从而得到从起点到终点的最短路径。
**使用Dijkstra算法**:
Dijkstra算法适合有权重的图,如果你的迷宫有特定的难度值(如距离或代价),你可以用它来寻找最低成本的路径。该算法从起点开始,逐步更新到每个节点的最短路径。
**相关问题--:**
1. 如何在MATLAB中表示一个二维迷宫矩阵?
2. Dijkstra算法与BFS相比,在什么情况下更适用?
3. 如果迷宫有复杂规则(如动态变化或随机生成),如何处理?
如果需要具体代码示例,请告诉我,我可以提供一个简化版的MATLAB代码示例。
相关问题
matlab迷宫最短路径算法
对于解决迷宫最短路径问题,可以使用图论中的最短路径算法,其中最常用的是Dijkstra算法和A*算法。在MATLAB中,可以使用以下步骤实现迷宫最短路径算法:
1. 创建迷宫地图:
首先,你需要创建一个表示迷宫的地图。可以使用矩阵来表示,其中0表示可通行的路径,1表示墙壁或障碍物。例如,一个3x3的迷宫可以表示为:
```matlab
maze = [0 1 0;
0 0 1;
1 0 0];
```
2. 构建图数据结构:
将迷宫地图转换为图数据结构,以便进行最短路径计算。你可以使用MATLAB的graph函数来创建一个图对象,并设置每个节点之间的连接关系。将可通行的路径作为节点,通过边连接相邻的节点。例如:
```matlab
G = graph;
[rows, cols] = size(maze);
for i = 1:rows
for j = 1:cols
if maze(i, j) == 0
node = (i - 1) * cols + j;
neighbors = [];
if i > 1 && maze(i-1, j) == 0
neighbors = [neighbors, node - cols];
end
if i < rows && maze(i+1, j) == 0
neighbors = [neighbors, node + cols];
end
if j > 1 && maze(i, j-1) == 0
neighbors = [neighbors, node - 1];
end
if j < cols && maze(i, j+1) == 0
neighbors = [neighbors, node + 1];
end
G = addedge(G, repmat(node, size(neighbors)), neighbors);
end
end
end
```
3. 计算最短路径:
使用Dijkstra算法或A*算法计算从起点到终点的最短路径。可以使用MATLAB的shortestpath函数来实现,它接受图对象和起点、终点作为输入,并返回最短路径。例如:
```matlab
startNode = 1; % 起点节点
endNode = rows * cols; % 终点节点
path = shortestpath(G, startNode, endNode);
```
这样,你就可以在MATLAB中实现迷宫最短路径算法了。记得适当处理特殊情况,比如无法达到终点或起点无效等。
matlab动态规划最短路径
在MATLAB中,可以使用动态规划算法来求解最短路径问题。动态规划是一种通过将问题分解为子问题并利用子问题的解来求解原问题的方法。
下面是使用动态规划算法求解最短路径的一般步骤:
1. 定义状态:将问题抽象为一个状态转移的过程。对于最短路径问题,可以将每个节点定义为一个状态。
2. 定义状态转移方程:根据问题的特点,定义状态之间的转移关系。对于最短路径问题,可以使用一个二维数组来表示节点之间的距离或权重。
3. 初始化:设置起始节点的距离为0,其他节点的距离为无穷大。
4. 迭代计算:通过迭代计算更新每个节点的最短距离,直到达到终止条件。在每次迭代中,根据状态转移方程更新节点的距离。
5. 回溯路径:在计算过程中,记录每个节点的前驱节点,最后根据前驱节点回溯得到最短路径。
下面是一个MATLAB示例代码,演示如何使用动态规划算法求解最短路径问题:
```matlab
function shortestPath = dynamicProgrammingShortestPath(graph, startNode, endNode)
numNodes = size(graph, 1);
distances = inf(1, numNodes);
distances(startNode) = 0;
predecessors = zeros(1, numNodes);
for i = 1:numNodes
for j = 1:numNodes
if graph(i, j) ~= 0
if distances(j) > distances(i) + graph(i, j)
distances(j) = distances(i) + graph(i, j);
predecessors(j) = i;
end
end
end
end
% 回溯路径
path = [endNode];
currentNode = endNode;
while currentNode ~= startNode
currentNode = predecessors(currentNode);
path = [currentNode, path];
end
shortestPath = path;
end
```
在上述代码中,`graph`是一个表示节点之间距离或权重的二维数组,`startNode`和`endNode`分别表示起始节点和终止节点。函数返回最短路径。
相关推荐
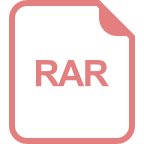












