编写程序输入学生成绩,统计并输出最高分和最低分,输入负数结束输入
时间: 2024-11-20 13:40:59 浏览: 22
编写一个简单的程序,通常用于命令行或控制台交互中,可以按照以下步骤进行:
1. 首先,创建一个变量来存储学生的成绩列表,初始化为空。
2. 使用while循环,提示用户输入成绩。如果输入的是负数,则跳出循环。
```python
scores = []
while True:
score = float(input("请输入学生分数(输入负数结束):"))
if score < 0:
break
scores.append(score)
```
3. 接下来,通过遍历成绩列表找到最高分和最低分。可以分别用一个变量(如max_score和min_score)记录它们的值,并在每次遇到新分数时更新。
```python
max_score = min_score = scores[0] if scores else None
for score in scores:
if score > max_score:
max_score = score
elif score < min_score:
min_score = score
```
4. 最后,输出最高分和最低分。
```python
print(f"最高分为:{max_score}")
print(f"最低分为:{min_score}")
```
相关问题
编写python程序,通过input函数录入几位同学成绩保存到列表score中,输入负分录入结束,然后计算并输出列表score中成绩在[80,90)区间的平均分以及所有成绩的最高分和最低分。(要求程序交互友好、足够强壮)
好的,下面是实现您需要的 Python 代码:
```python
score = []
while True:
s = float(input("请输入同学的成绩:"))
if s < 0:
break
score.append(s)
count = len(score)
if count == 0:
print("没有输入任何成绩!")
else:
sum_80_90 = 0
max_score = min_score = score[0]
for s in score:
if s >= 80 and s < 90:
sum_80_90 += s
if s > max_score:
max_score = s
if s < min_score:
min_score = s
if sum_80_90 == 0:
print("成绩在[80,90)区间的同学不存在!")
else:
avg_80_90 = sum_80_90 / count
print("成绩在[80,90)区间的同学的平均分为:", avg_80_90)
print("所有同学的最高分为:", max_score)
print("所有同学的最低分为:", min_score)
```
这个程序会先让用户输入同学的成绩,直到输入负数为止。然后会计算列表中成绩在[80,90)区间的平均分以及所有成绩的最高分和最低分,并输出结果。如果没有输入任何成绩或者成绩在[80,90)区间的同学不存在,也会有相应的提示信息。
python编写一段程序,根据分数进行升序排序后,输出学生的数量,成绩的最低分和最高分,成绩为前n名和后n名的同学姓名(n为非负数,当n大于数据行数时,按实际行数输出),以及平均成绩(保留小数点后2位)。
好的,这里是Python程序的实现:
```python
# 读取分数数据
scores = []
with open('scores.txt') as f:
for line in f:
name, score = line.strip().split()
scores.append((name, int(score)))
# 对分数进行升序排序
scores.sort(key=lambda x: x[1])
# 统计学生数量、最低分、最高分和平均分
num_students = len(scores)
min_score = scores[0][1]
max_score = scores[-1][1]
avg_score = sum([score for _, score in scores]) / num_students
# 输出结果
print(f"学生数量:{num_students}")
print(f"最低分:{min_score}")
print(f"最高分:{max_score}")
print(f"平均分:{avg_score:.2f}")
n = int(input("请输入n的值:"))
# 输出前n名同学的姓名
print(f"前{n}名同学的姓名:")
for name, score in scores[:n]:
print(name)
# 输出后n名同学的姓名
print(f"后{n}名同学的姓名:")
for name, score in scores[-n:]:
print(name)
```
程序的输入是一个文件 `scores.txt`,每行包含一个学生的姓名和分数,中间用空格隔开。例如:
```
张三 90
李四 80
王五 85
```
程序的输出包括学生数量、最低分、最高分、平均分、前n名同学的姓名、后n名同学的姓名。其中,n由用户输入。
阅读全文
相关推荐
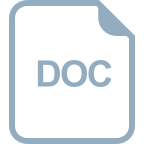
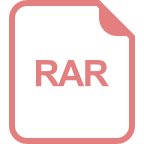
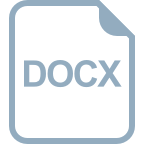
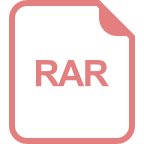
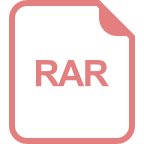
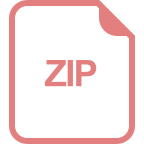
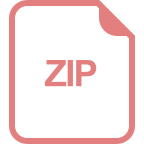
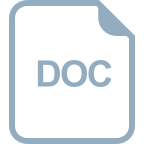



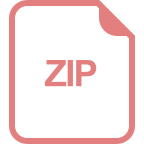
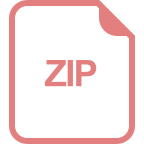
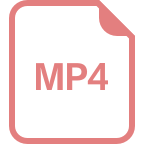