请使用逻辑斯蒂回归训练手写数字数据集MNIST,请划分数据集成为训练集和测试集并按照8:2的比例进行划分,请提供代码和预测结果。
时间: 2024-10-07 18:06:29 浏览: 49
在Python中,我们可以使用scikit-learn库来处理逻辑斯蒂回归(Logistic Regression)模型,并结合MNIST手写数字数据集。以下是大致步骤:
首先,确保已经安装了所需的库:
```bash
pip install numpy pandas scikit-learn matplotlib
```
然后,导入必要的模块并加载MNIST数据:
```python
import numpy as np
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load the MNIST dataset
mnist = fetch_openml('mnist_784', version=1)
```
接下来,我们对数据进行预处理,将图像转换为一维数组,并划分训练集和测试集(80%训练,20%测试):
```python
X = mnist.data / 255.
y = mnist.target
# Split into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
现在,我们可以创建逻辑斯蒂回归模型并训练它:
```python
model = LogisticRegression()
model.fit(X_train, y_train)
```
训练完成后,我们可以对测试集进行预测,并计算准确率:
```python
y_pred = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy on test set: {accuracy * 100:.2f}%")
```
由于完整代码和实际运行需要环境支持,这里只给出了基本框架。运行上述代码时,你需要在一个有适当库环境的环境中运行,例如Jupyter Notebook或本地Python环境。
阅读全文
相关推荐
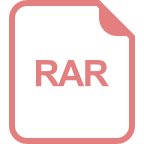
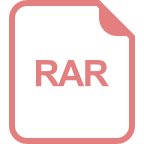
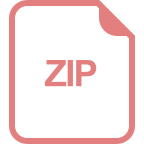
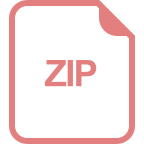
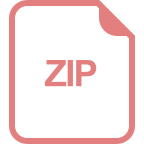
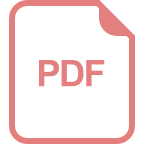
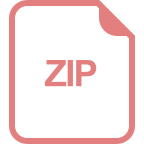
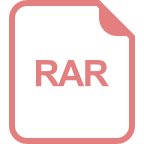
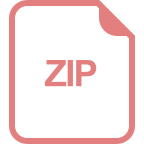
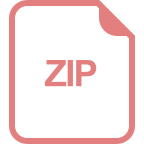
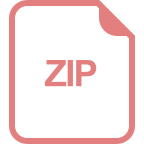
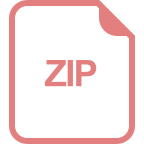
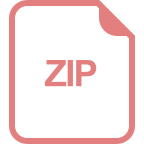
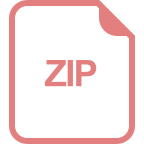
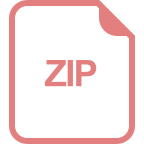
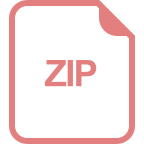