在一个带头结点的单链表的指定位置location,增加一个新的元素x,若给定的位置不合法则提示插入失败。c语言
时间: 2024-09-25 07:10:13 浏览: 30
在C语言中,要在单链表的指定位置`location`插入新元素`x`,通常需要遵循以下步骤:
1. **检查有效性**:
- 首先,确认`location`是否有效。如果它小于0或大于链表的实际长度(即头节点之后的节点数),那么插入位置不合法,此时可以返回错误信息,例如 `printf("Insertion failed: Invalid location.\n");`。
2. **定位插入位置**:
- 使用循环从头节点开始遍历链表,找到第`location`个节点。当计数达到`location-1`时,当前节点就是前一个节点。
3. **插入新节点**:
- 创建一个新的`struct Node`并初始化它的数据域为`x`。
- 将新节点的`next`指针设置为当前节点的`next`,以便将当前节点指向新节点。
- 如果`location`是第一个节点(即头节点),则直接将新节点设为头节点。
4. **返回结果**:
- 插入操作成功后,无需特别通知,因为新的链表结构已经更新了。
以下是简单的伪代码示例:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
void insertAtLocation(Node** head, int x, int location) {
if (location <= 0) {
printf("Insertion failed: Invalid location.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = x;
newNode->next = *head;
Node* current = *head;
for (int i = 1; i < location && current != NULL; ++i) {
current = current->next;
}
if (current == NULL) {
printf("Insertion failed: Location out of range.\n");
free(newNode);
return;
}
current->next = newNode;
}
```
相关推荐
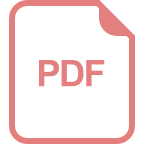
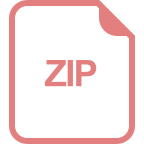














