React There is a chart instance already initialized on the dom
时间: 2023-10-12 17:03:53 浏览: 142
If you have already initialized a chart instance in a React application, you can access it using a ref. Here's an example:
```jsx
import React, { useRef, useEffect } from 'react';
import Chart from 'chart.js';
const MyChartComponent = () => {
const chartRef = useRef(null);
useEffect(() => {
// Access the chart instance using the ref
const chartInstance = chartRef.current;
// You can modify or interact with the chart instance here
// Clean up when the component unmounts
return () => {
// Destroy the chart instance if needed
if (chartInstance) {
chartInstance.destroy();
}
};
}, []);
return <canvas ref={chartRef} />;
};
export default MyChartComponent;
```
In this example, the `chartRef` is used to access the chart instance. You can modify or interact with the chart instance within the `useEffect` hook. Don't forget to clean up the chart instance when the component unmounts to avoid memory leaks.
阅读全文
相关推荐
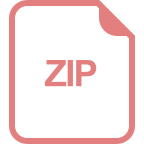
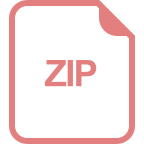
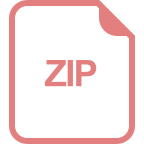


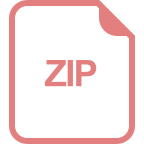
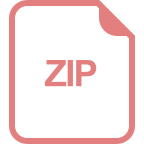
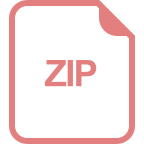
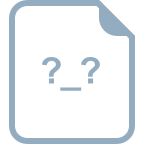
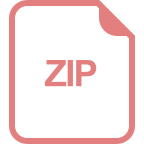
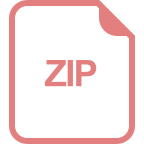
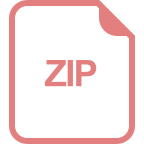
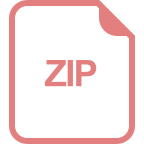
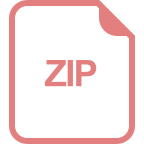
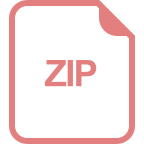
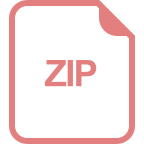
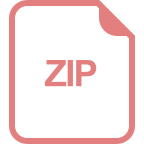
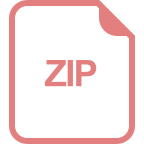
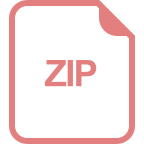