分别使用XML和注解的AOP方式实现如下所示的运行结果‘ (前置通知)佣人准备笔墨纸砚 (环绕前)唐伯虎润笔 唐伯虎画画 (环绕后)唐伯虎收笔 (返回通知)装裱 (后置通知)佣人收拾笔墨纸
时间: 2024-12-23 18:12:31 浏览: 16
在Spring框架中,Aspect-Oriented Programming (AOP) 可以通过多种方式实现切面编程,其中一种常见的就是使用XML配置和注解。这里我们将分别解释这两种方式:
1. **使用XML配置**:
- 首先,你需要在Spring的`beans.xml`文件中定义切面(Aspect),比如:
```xml
<aop:config>
<aop:aspect id="paintingAspect">
<!-- 使用<before>标签表示前置通知 -->
<aop:before method="prepareMaterials" pointcut="@annotation(Preparation)" />
<!-- 使用<around>标签表示环绕通知 -->
<aop:around method="aroundAdvice" pointcut="@annotation(Paint)" target="execution(* com.example.Drawing.*(..))" />
<!-- 使用<after-return>标签表示返回后通知 -->
<aop:after-return method="cleanUp" pointcut="@annotation(ReturnNotification)" return-object="result" />
<!-- 使用<after>标签表示后置通知 -->
<aop:after method="packaging" pointcut="@annotation(PostProcessing)" />
</aop:aspect>
<!-- 定义切点和通知方法的bean -->
<bean id="preparation" class="com.example.Preparation" />
<bean id="paint" class="com.example.Paint" />
<bean id="returnNotification" class="com.example.ReturnNotification" />
<bean id="postProcessing" class="com.example.PostProcessing" />
</aop:config>
```
- 在各个自定义注解如`@Preparation`, `@Paint`, `@ReturnNotification`, 和 `@PostProcessing` 中,你需要提供具体的执行逻辑。
2. **使用注解实现**:
- 创建带有注解的切点表达式:
```java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Preparation {
// 添加额外的信息如果需要
}
// 类似地创建其他注解...
```
- 在切面类中,利用Spring AOP注解处理器处理这些注解:
```java
@Aspect
@Component
public class PaintingAspect {
@Before("@annotation(com.example.Preparation)")
public void prepareMaterials(Preparation preparationAnnotation) {
System.out.println("佣人准备笔墨纸砚");
}
@Around("@annotation(com.example.Paint)")
public Object aroundAdvice(ProceedingJoinPoint joinPoint, Paint paintAnnotation) throws Throwable {
// ...执行环绕通知逻辑...
Object result = joinPoint.proceed();
return result;
}
// 其他通知...
}
```
无论哪种方式,最后的结果都是在特定方法执行前后插入相应的逻辑。记得导入必要的Spring AOP依赖,并按照实际项目结构调整类路径和命名空间。
阅读全文
相关推荐










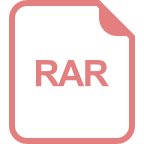







