蛮力法0/1背包问题伪代码
时间: 2023-11-26 20:45:21 浏览: 122
蛮力法(Brute Force)是一种暴力枚举的算法,它通过枚举所有可能的解来寻找最优解。对于0/1背包问题,蛮力法的伪代码如下:
1. 初始化最大价值为0
2. 枚举所有可能的物品组合:
a. 对于每个物品,可以选择放入或不放入背包
b. 对于每种组合,计算其总重量和总价值
c. 如果总重量不超过背包容量且总价值大于当前最大价值,则更新最大价值
3. 返回最大价值
需要注意的是,蛮力法的时间复杂度为O(2^n),其中n为物品数量,因此对于大规模的问题,蛮力法的效率非常低下。
相关问题
蛮力法0/1背包伪代码
蛮力法(Brute Force)是一种简单直接的解决问题的方法,它通过穷举所有可能的解来求解问题。以下是0/1背包问题的蛮力法伪代码:
```
function bruteForceKnapsack(weights, values, capacity):
n = length(weights) // 物品数量
max_value = 0 // 最大价值
best_selection = [] // 最佳选择
// 遍历所有可能的选择
for i from 0 to (2^n - 1):
current_weight = 0 // 当前选择的总重量
current_value = 0 // 当前选择的总价值
current_selection = [] // 当前选择的物品
// 将i转换为二进制表示,表示物品的选择情况
for j from 0 to (n - 1):
if (i & (1 << j)) != 0:
current_weight += weights[j]
current_value += values[j]
current_selection.append(j)
// 如果当前选择的总重量不超过背包容量,并且总价值更大,则更新最大价值和最佳选择
if current_weight <= capacity and current_value > max_value:
max_value = current_value
best_selection = current_selection
return max_value, best_selection
```
背包问题蛮力法的伪代码
背包问题是一个经典的组合优化问题,蛮力法是一种解决该问题的简单但效率较低的方法。以下是背包问题蛮力法的伪代码:
```
function bruteForceKnapsack(weights, values, capacity):
n = length(weights) // 物品数量
max_value = 0 // 最大价值
best_combination = [] // 最佳组合
// 遍历所有可能的组合
for i from 0 to (2^n - 1):
current_weight = 0 // 当前组合的总重量
current_value = 0 // 当前组合的总价值
current_combination = [] // 当前组合的物品索引
// 将i转换为二进制表示,并根据二进制位决定是否选择对应物品
for j from 0 to (n - 1):
if (i & (1 << j)) != 0:
current_weight += weights[j]
current_value += values[j]
current_combination.append(j)
// 如果当前组合的总重量不超过背包容量,并且总价值更大,则更新最大价值和最佳组合
if current_weight <= capacity and current_value > max_value:
max_value = current_value
best_combination = current_combination
return max_value, best_combination
```
阅读全文
相关推荐
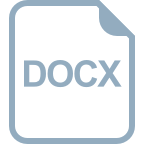
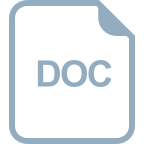

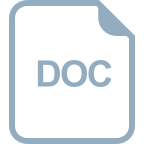
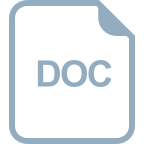

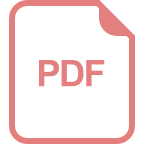
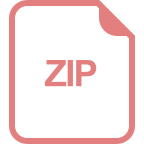