String转map
时间: 2023-08-19 11:15:05 浏览: 133
在Java中,可以使用以下方法将String转换为Map类型:
方法一:使用自定义的方法getValue(String param)。这个方法会将传入的字符串按照特定的规则解析成Map类型的数据。具体的实现代码如下:
```java
public static Map<String, Object> getValue(String param) {
Map<String, Object> map = new HashMap<>();
String str = "";
String key = "";
Object value = "";
char[] charList = param.toCharArray();
boolean valueBegin = false;
for (int i = 0; i < charList.length; i++) {
char c = charList[i];
if (c == '{') {
if (valueBegin == true) {
value = getValue(param.substring(i, param.length()));
i = param.indexOf('}', i) + 1;
map.put(key, value);
}
} else if (c == '=') {
valueBegin = true;
key = str;
str = "";
} else if (c == ',') {
valueBegin = false;
value = str;
str = "";
map.put(key, value);
} else if (c == '}') {
if (str != "") {
value = str;
}
map.put(key, value);
return map;
} else if (c != ' ') {
str += c;
}
}
return map;
}
```
方法二:使用自定义的方法mapStringToMap(String str)。这个方法会将传入的Map字符串解析成Map类型的数据。具体的实现代码如下:
```java
public static Map<String, String> mapStringToMap(String str) {
str = str.substring(1, str.length() - 1);
String[] strs = str.split(",");
Map<String, String> map = new HashMap<String, String>();
for (String string : strs) {
String key = string.split("=")[0];
String value = string.split("=")[1];
// 去掉头部空格
String key1 = key.trim();
String value1 = value.trim();
map.put(key1, value1);
}
return map;
}
```
使用这两个方法中的任意一个,你可以将String类型的数据转换为Map类型。例如:
```java
Map<String, Object> map = new HashMap<String, Object>();
map.put("aaa", 11);
map.put("bbb", 22);
map.put("ccc", 33);
map.put("ddd", 44);
String string = map.toString();
System.out.println("map转String:" + string);
Map<String, Object> stringToMap = getValue(string);
for (Object a : stringToMap.keySet()) {
System.out.println(stringToMap.get(a).toString());
}
```
这段代码会将Map类型的数据转换为String类型,并通过getValue方法将其再转换回Map类型,并打印出Map中的值。
阅读全文
相关推荐
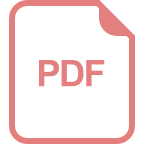















