如何在 FastAPI 应用中添加异步数据库操作?
时间: 2024-09-09 22:01:05 浏览: 86
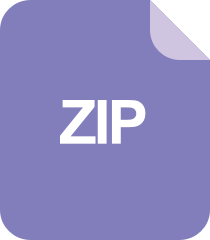
Python运维管理系统源代码,基于fastapi异步框架

在FastAPI中添加异步数据库操作,首先需要确保你有一个异步的数据库客户端。FastAPI是基于Starlette和Pydantic构建的,同时支持异步处理。为了实现异步数据库操作,你可以使用如`asyncio`库来处理异步代码,以及`aiomysql`或`aiopg`等支持异步操作的数据库驱动,对应于MySQL和PostgreSQL数据库。
下面是使用异步数据库操作的一个基本步骤:
1. 安装异步数据库驱动:例如,对于PostgreSQL,你可以使用`aiopg`。
```
pip install aiopg
```
2. 在FastAPI应用中创建异步数据库连接。这通常通过在依赖项中定义一个异步函数来实现,该函数负责建立连接。
```python
import aiopg
from fastapi import FastAPI, Depends
async def create_db_pool():
dsn = 'dbname=test user=test password=test host=127.0.0.1'
pool = await aiopg.create_pool(dsn)
return pool
app = FastAPI()
@app.on_event("startup")
async def startup():
app.state.pool = await create_db_pool()
@app.on_event("shutdown")
async def shutdown():
app.state.pool.close()
await app.state.pool.wait_closed()
```
3. 定义异步数据库操作函数。这些函数将会被用作路由操作中的依赖项。
```python
async def get_heroes(db_pool):
async with db_pool.acquire() as conn:
async with conn.cursor() as cursor:
await cursor.execute("SELECT * FROM heros")
return await cursor.fetchall()
```
4. 在FastAPI路由中使用这些异步函数。可以通过`Depends`函数来依赖这些异步操作。
```python
@app.get("/heroes")
async def get_heroes_list(db_pool=Depends(get_heroes)):
return db_pool
```
这样,当你访问FastAPI应用中的`/heroes`路径时,它将执行异步函数`get_heroes`来从数据库获取英雄列表。
阅读全文
相关推荐
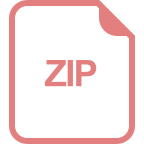
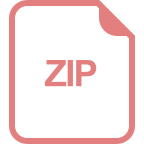
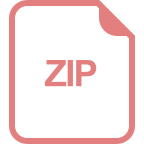
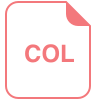
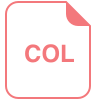
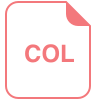
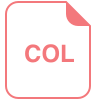
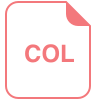
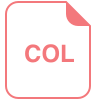
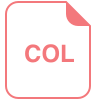
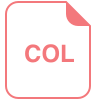
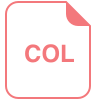
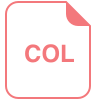
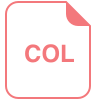
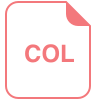
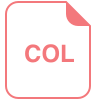
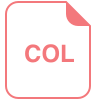
