C#如何防止操作UI控件对其他线程精度产生影响、
时间: 2024-09-21 07:07:10 浏览: 41
在C#中,由于UI线程负责控制应用程序的用户界面更新,因此直接在非UI线程上修改UI控件可能会引发异常或导致UI阻塞。为了防止这种影响,你可以采用以下几种策略:
1. **使用`Dispatcher`委托**:这是最推荐的做法,尤其是在Windows Forms或WPF应用中。所有从非UI线程向UI发送的消息都需要通过`Dispatcher`进行调度。例如:
```csharp
private void NonUiThreadWork()
{
Dispatcher.Invoke(new Action(() => myButton.Text = "Updating UI"));
}
// 或者更简洁的形式,如果你正在使用async/await:
private async void NonUiThreadWorkAsync()
{
await Task.Run(() => { myButton.Text = "Updating UI"; });
}
```
2. **`BeginInvoke`和`EndInvoke`**:这两个方法也可以用来异步地在UI线程上执行代码。例如:
```csharp
button.BeginInvoke(new Action(() => button.Text = "Updating UI"));
```
3. **`Task.Run`配合`await`**:结合async/await,可以在后台线程完成任务后再更新UI,同时让UI保持响应:
```csharp
await Task.Run(() => UpdateMyControl());
private void UpdateMyControl()
{
this.Dispatcher.Invoke(() => MyControl.Text = "New Value");
}
```
4. **使用`BlockingCollection`+`BackgroundWorker`**:虽然不是首选,但若你需要在多个循环中异步更新UI,可以考虑使用`BlockingCollection`来同步线程:
```csharp
BackgroundWorker worker = new BackgroundWorker();
worker.DoWork += (s, e) => UpdateUI(e);
// ...
public void UpdateUI(DoWorkEventArgs e)
{
var collection = new BlockingCollection<string>();
// 更新UI的异步任务
Task.Factory.StartNew(() => UpdateTextAsync(collection));
// 线程安全收集结果并显示
collection.GetConsumingEnumerable().ToList().ForEach(text => Invoke((Action)(() => TextBlock.Text = text)));
}
private async Task UpdateTextAsync(BlockingCollection<string> collection)
{
for (string value in valuesToProcess)
{
collection.Add(value);
await Task.Delay(100); // 模拟延迟操作
}
}
```
总之,关键在于确保所有的UI更改都在UI线程上执行,以维护应用程序的稳定性和用户体验。
相关推荐
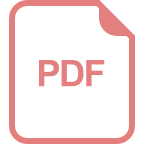
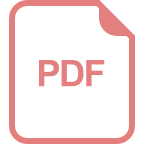
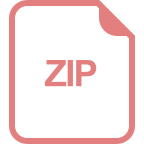















