C++ expand函数
时间: 2024-10-26 15:01:16 浏览: 9
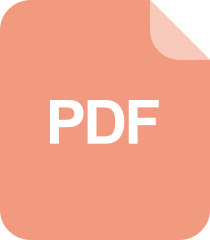
C、C++函数集(速查).pdf
在C++中,并不存在直接称为"expand"的函数。不过,如果你是指字符串的`std::string::find`、`std::string::substr`或者`std::string::replace`等操作,它们可以间接地“扩展”或修改字符串。
例如,`find`函数可以在字符串中查找某个子串第一次出现的位置,如果找到则返回位置,否则返回`npos`;`substr`函数可以根据起始位置提取子串;而`replace`函数则可以替换指定位置的字符或子串。
如果你想实现类似于字符串展开的功能,比如将特定字符或子串替换为多次复制,你可以使用`substr`配合循环来达到目的,如:
```cpp
void stringExpand(std::string& str, const std::string& target, int count) {
size_t pos = 0;
while (pos != str.npos && count > 0) {
pos = str.find(target, pos);
if (pos != str.npos) {
str.replace(pos, target.size(), target);
pos += target.size(); // 移动查找起点
count--;
}
}
}
```
在这个例子中,`stringExpand`函数会查找并替换目标子串到指定次数。
阅读全文
相关推荐
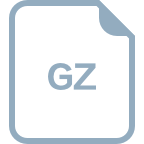
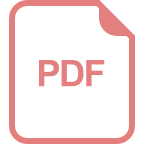















