不使用messagebox,使用dev中的gridcontrol控件中的gridview显示,该如何修改代码
时间: 2024-04-15 16:30:34 浏览: 147
要在 Dev 中的 GridControl 控件中的 GridView 显示学生信息,您需要进行以下修改:
1. 添加 `using DevExpress.XtraGrid;` 和 `using DevExpress.XtraGrid.Views.Grid;` 引用。
2. 将 `Program` 类修改为继承自 `DevExpress.XtraEditors.XtraForm`。
3. 在窗体上放置一个 `GridControl` 控件,并将其 Dock 属性设置为 `Fill`。
4. 将原来的 `MessageBox.Show` 改为使用 `gridView.SetRowCellValue` 方法将学生信息添加到 GridView 中。
以下是修改后的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Text.RegularExpressions;
using System.Windows.Forms;
using DevExpress.XtraGrid;
using DevExpress.XtraGrid.Views.Grid;
public class UserInfo
{
public string Name { get; set; }
public string Age { get; set; }
public string Gender { get; set; }
public string Phone { get; set; }
public string Email { get; set; }
}
public class Program : DevExpress.XtraEditors.XtraForm
{
private GridControl gridControl;
private GridView gridView;
private Button extractButton;
public Program()
{
gridControl = new GridControl();
gridView = new GridView();
extractButton = new Button();
extractButton.Text = "提取用户信息";
extractButton.Click += ExtractButton_Click;
gridView.GridControl = gridControl;
gridControl.Dock = DockStyle.Fill;
gridControl.ViewCollection.Add(gridView);
Controls.Add(gridControl);
Controls.Add(extractButton);
}
private void ExtractButton_Click(object sender, EventArgs e)
{
string filePath = "path/to/your/txt/file.txt";
UserInfo userInfo = ExtractUserInfo(filePath);
gridView.SetRowCellValue(0, "Name", userInfo.Name);
gridView.SetRowCellValue(0, "Age", userInfo.Age);
gridView.SetRowCellValue(0, "Gender", userInfo.Gender);
gridView.SetRowCellValue(0, "Phone", userInfo.Phone);
gridView.SetRowCellValue(0, "Email", userInfo.Email);
}
private UserInfo ExtractUserInfo(string filePath)
{
string content = File.ReadAllText(filePath);
string name = GetMatchValue(content, @"姓名:([\w\s]+)");
string age = GetMatchValue(content, @"年龄:(\d+)");
string gender = GetMatchValue(content, @"性别:([\w\s]+)");
string phone = GetMatchValue(content, @"电话:(\d+)");
string email = GetMatchValue(content, @"邮箱:([\w\.]+@[\w\.]+)");
UserInfo userInfo = new UserInfo
{
Name = name,
Age = age,
Gender = gender,
Phone = phone,
Email = email
};
return userInfo;
}
private string GetMatchValue(string content, string pattern)
{
Match match = Regex.Match(content, pattern);
return match.Success ? match.Groups[1].Value : null;
}
public static void Main(string[] args)
{
Application.Run(new Program());
}
}
```
这个示例代码中,我们添加了 `DevExpress.XtraGrid` 和 `DevExpress.XtraGrid.Views.Grid` 的引用,并使用 `GridControl` 和 `GridView` 控件来显示学生信息。
在点击按钮后,我们使用 `gridView.SetRowCellValue` 方法将学生信息添加到 GridView 中的第一行。请确保将 `filePath` 替换为实际的文件路径。运行程序后,点击按钮即可触发提取操作,并将学生信息显示在 GridView 中的第一行中。
阅读全文
相关推荐
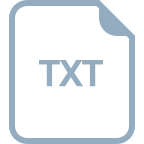
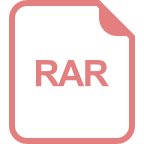
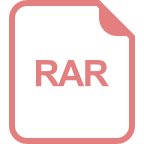
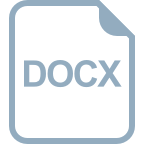
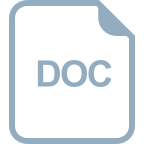
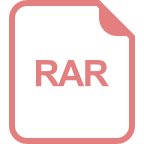
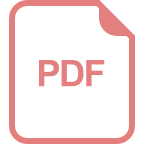
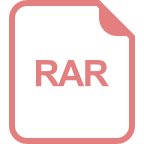
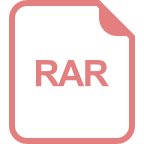
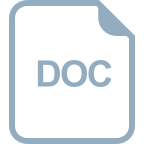
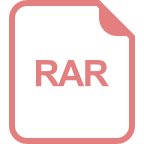
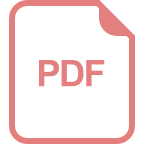
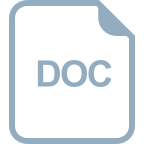

