mqtt断网后在如何在connlost回调函数中重新连接mqtt
时间: 2023-12-22 21:34:48 浏览: 190
在 MQTT 的 connlost 回调函数中重新连接 MQTT 服务器可以通过以下步骤实现:
1. 在主程序中,定义一个全局的 MQTT 客户端结构体变量,用于保存 MQTT 连接的状态和参数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <MQTTClient.h>
volatile MQTTClient_deliveryToken deliveredtoken;
typedef struct {
MQTTClient client;
char* broker_address;
char* client_id;
char* username;
char* password;
} MQTTConnection;
MQTTConnection mqtt_connection;
```
2. 在主程序中,定义一个函数用于连接 MQTT 服务器,并在断网后重新连接。
```c
int connectToMQTTServer() {
MQTTClient_connectOptions conn_opts = MQTTClient_connectOptions_initializer;
conn_opts.keepAliveInterval = 20;
conn_opts.cleansession = 1;
int rc;
if ((rc = MQTTClient_create(&mqtt_connection.client, mqtt_connection.broker_address, mqtt_connection.client_id,
MQTTCLIENT_PERSISTENCE_NONE, NULL)) != MQTTCLIENT_SUCCESS) {
printf("Failed to create MQTT client, return code %d\n", rc);
return rc;
}
conn_opts.username = mqtt_connection.username;
conn_opts.password = mqtt_connection.password;
if ((rc = MQTTClient_setCallbacks(mqtt_connection.client, NULL, connlost, msgarrvd, delivered)) != MQTTCLIENT_SUCCESS) {
printf("Failed to set callbacks, return code %d\n", rc);
return rc;
}
if ((rc = MQTTClient_connect(mqtt_connection.client, &conn_opts)) != MQTTCLIENT_SUCCESS) {
printf("Failed to connect to MQTT server, return code %d\n", rc);
return rc;
}
printf("Connected to MQTT server\n");
return MQTTCLIENT_SUCCESS;
}
```
3. 在主程序中,定义 connlost 回调函数,用于处理 MQTT 连接断开的情况。
```c
void connlost(void* context, char* cause) {
printf("\nConnection lost\n");
printf("Reconnecting to MQTT server...\n");
int rc;
do {
rc = connectToMQTTServer();
if (rc != MQTTCLIENT_SUCCESS) {
printf("Failed to reconnect to MQTT server, return code %d. Retrying in 5 seconds...\n", rc);
sleep(5);
}
} while (rc != MQTTCLIENT_SUCCESS);
}
```
在 connlost 回调函数中,我们首先打印出连接断开的消息,并通过 connectToMQTTServer() 函数重新连接 MQTT 服务器。如果重新连接失败,我们会等待 5 秒钟后再次尝试连接,直到连接成功。
4. 在主程序中,使用 MQTTClient_setCallbacks() 函数将 connlost 回调函数设置为 MQTT 客户端的回调函数。
```c
int main() {
// 初始化 mqtt_connection 结构体变量的属性(broker_address、client_id、username、password)
// 设置 connlost 回调函数
MQTTClient_setCallbacks(mqtt_connection.client, NULL, connlost, msgarrvd, delivered);
// 连接到 MQTT 服务器
int rc = connectToMQTTServer();
// ...
return 0;
}
```
在主程序中,我们使用 MQTTClient_setCallbacks() 函数将 connlost 回调函数设置为 MQTT 客户端的回调函数之一。
当 MQTT 连接断开时,系统会自动调用 connlost 回调函数,并在该回调函数中重新连接 MQTT 服务器。
请注意,上述代码只是一个简单示例,并不包含完整的错误处理和其他细节。在实际使用中,您可能需要根据具体情况进行修改和扩展。
希望这能帮助您重新连接 MQTT 服务器!如果还有其他问题,请随时提问。
阅读全文
相关推荐
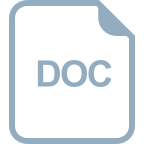
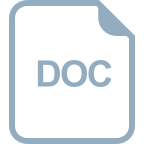
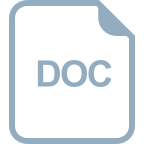
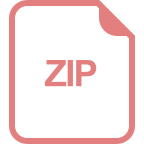
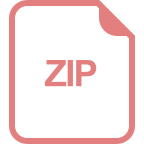
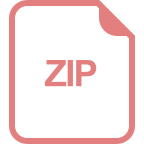
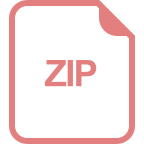
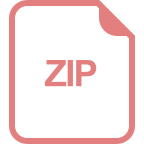
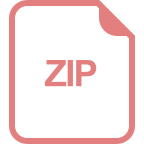
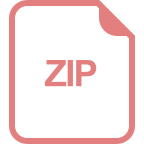
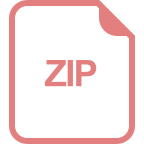
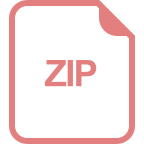
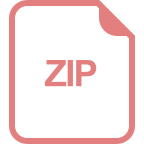
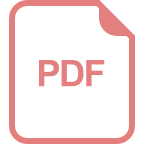
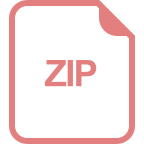
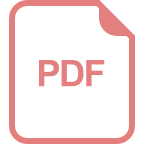