用python画一个时钟表盘
时间: 2024-09-28 13:04:07 浏览: 29
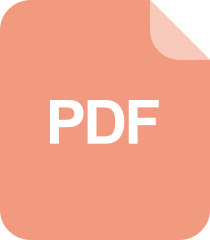
python使用turtle库绘制时钟

在Python中,我们可以使用PIL(Pillow)库来绘制一个简单的时钟表盘。下面是一个基本的示例,它会创建一个圆形并添加数字和指针:
```python
from PIL import Image, ImageDraw, ImageFont
# 创建一个新的白色背景图片
img = Image.new('RGB', (400, 400), 'white')
draw = ImageDraw.Draw(img)
# 设置字体大小和颜色
font_size = 80
font_color = 'black'
# 定义圆心、半径和颜色
center = (200, 200)
radius = img.size[0] // 2 - 50
hour_color = 'gray'
minute_color = 'red'
# 画圆
draw.ellipse((center[0] - radius, center[1] - radius, center[0] + radius, center[1] + radius), fill=hour_color)
# 加上小时指针
hour_angle_start = 360 / 12 * int(6) # 假设当前时间是下午6点
hour_angle_end = hour_angle_start + 30 # 指针长度约为30度
hour_line = draw.line((center[0], center[1] - 50, center[0] + int(radius * (hour_angle_end - hour_angle_start) / 360), center[1] + 50),
fill=hour_color, width=30)
# 加上分钟指针
minute_angle_start = 360 / 60 * int(30) # 假设当前时间是30分钟
minute_angle_end = minute_angle_start + 6 # 指针长度约为6度
minute_line = draw.line((center[0], center[1] - 50, center[0] + int(radius * (minute_angle_end - minute_angle_start) / 360), center[1] + 50),
fill=minute_color, width=15)
# 添加数字标签
font = ImageFont.truetype('arial.ttf', font_size) # 使用系统可用的字体
for i in range(1, 13):
degree = 360 / 12 * i
x = center[0] + radius * (math.cos(math.radians(degree)) * math.sin(90 - font_size))
y = center[1] + radius * (math.sin(math.radians(degree)) * math.sin(90 - font_size))
draw.text((x, y), str(i), font=font, fill=font_color)
# 显示图像
img.show()
```
这个例子假设你知道如何安装PIL库,并且电脑上有Arial字体文件。运行这段代码会弹出一个显示时钟的窗口。
阅读全文
相关推荐
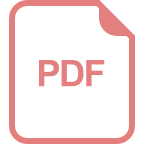
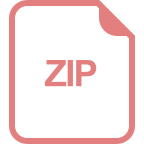


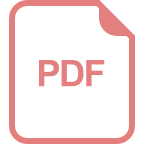
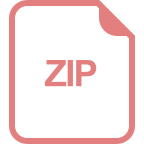
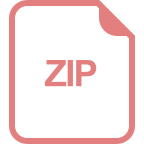
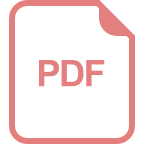
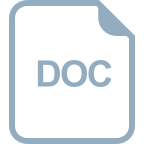
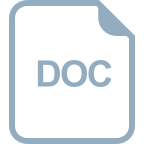
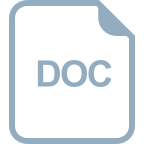
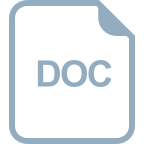
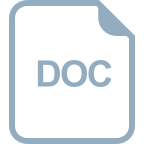




