c语言通讯录管理系统实现对通讯录信息的录入、查找、修改、删除等相关功能。
时间: 2024-12-16 19:18:38 浏览: 7
在C语言中实现一个通讯录管理系统,可以使用结构体来存储联系人信息,然后设计相应的函数来实现录入、查找、修改和删除等操作。以下是简单的流程说明:
1. 定义一个结构体表示联系人:
```c
struct Contact {
char name[50];
char phone_number[20];
char email[50];
};
```
2. 创建用于管理联系人的全局变量或动态内存池:
```c
struct Contact contacts[100]; // 假设最多有100个联系人
int contact_count = 0; // 记录当前联系人数
```
3. 录入联系人信息:
```c
void add_contact(struct Contact* new_contact) {
if (contact_count < 100) {
contacts[contact_count++] = *new_contact;
printf("Contact added successfully.\n");
} else {
printf("Contact list is full.\n");
}
}
```
4. 查找联系人:
```c
bool find_contact(const char* search_name, struct Contact* found_contact) {
for (int i = 0; i < contact_count; i++) {
if (strcmp(contacts[i].name, search_name) == 0) {
*found_contact = contacts[i];
return true;
}
}
return false;
}
```
5. 修改联系人信息:
```c
void update_contact(const char* old_name, struct Contact* updated_info) {
bool found = false;
for (int i = 0; i < contact_count; i++) {
if (strcmp(contacts[i].name, old_name) == 0) {
found = true;
memcpy(&contacts[i], updated_info, sizeof(struct Contact));
break;
}
}
if (found) {
printf("Contact updated.\n");
} else {
printf("Contact not found.\n");
}
}
```
6. 删除联系人:
```c
void delete_contact(const char* name_to_remove) {
for (int i = 0; i < contact_count - 1; i++) {
if (strcmp(contacts[i].name, name_to_remove) == 0) {
memmove(&contacts[i], &contacts[i + 1], (contact_count - i - 1) * sizeof(struct Contact));
contact_count--;
printf("Contact deleted.\n");
break;
}
}
}
```
以上就是基本的通讯录管理系统的实现,需要注意的是,这个示例没有考虑错误处理和内存管理的细节。
阅读全文
相关推荐
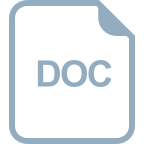
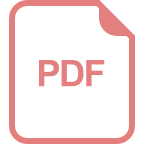
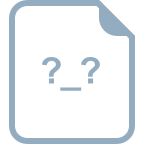















