c++实现:删除ubuntu指定路径下超过最近10天保存的log文件
时间: 2024-09-28 14:15:33 浏览: 29
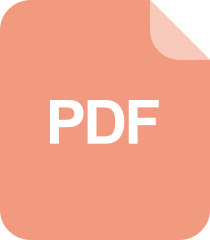
基于C++实现读取指定路径文件
在Ubuntu系统上,你可以使用C++编写一个简单的脚本来实现这个需求。这里我们将使用`std::filesystem`库来处理文件操作,并配合`time.h`库来计算时间差。注意,你需要先安装`libstdc++6-dev`(或者其他包含`std::filesystem`支持的库)才能编译。
```cpp
#include <iostream>
#include <filesystem>
#include <ctime>
// 函数用来检查文件是否超过10天
bool isFileOld(const std::filesystem::path& path, time_t days_threshold = 10) {
struct stat file_info;
if (stat(path.c_str(), &file_info) != 0) {
std::cerr << "Error: Could not get file info for " << path.string() << std::endl;
return false;
}
// 获取文件创建时间(以秒为单位)
auto file_age = time(nullptr) - file_info.st_ctime;
// 检查文件是否大于阈值
return file_age > days_threshold * 24 * 60 * 60; // 一天有86400秒
}
int main() {
std::string directory_path = "/path/to/your/log/directory"; // 替换为你需要检查的目录
int days_to_keep = 10; // 文件保留天数
for (const auto& entry : std::filesystem::directory_iterator(directory_path)) {
if (entry.is_regular_file()) { // 只处理普通文件
if (isFileOld(entry.path(), days_to_keep)) {
std::cout << "Deleting old log file: " << entry.path().filename() << std::endl;
// 删除文件,这里只是打印出来,实际操作请谨慎,可能需要管理员权限
std::filesystem::remove(entry.path());
}
}
}
return 0;
}
```
阅读全文
相关推荐
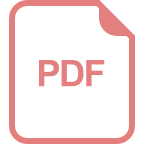
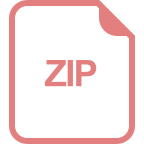
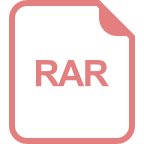
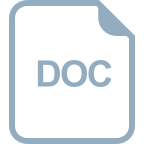
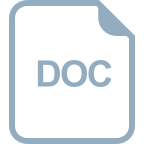
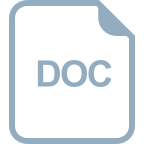
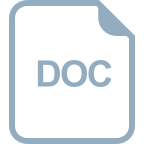
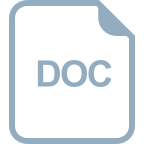
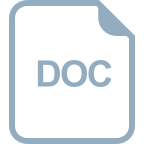
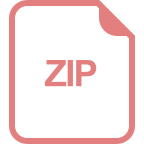
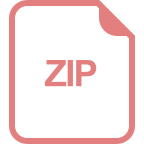
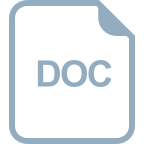
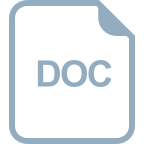
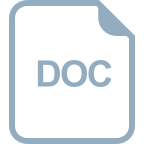
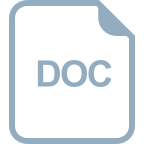
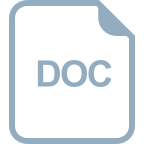