如何在Uni-app中实现输入框内检测并高亮显示敏感词的功能?
时间: 2024-12-12 07:27:00 浏览: 5
在Uni-app中实现输入框内检测并高亮显示敏感词的功能,你可以按照以下步骤操作:
1. **安装依赖库**:首先需要安装一个文本处理的第三方库,如`vue-sensitive-word-filter`,可以使用npm或yarn进行安装:
```bash
npm install vue-sensitive-word-filter 或者 yarn add vue-sensitive-word-filter
```
2. **创建组件**:在你的Vue组件中引入这个库,并在模板中集成一个输入框(Input)和敏感词过滤器:
```html
<template>
<div>
<input v-model="searchText" @input="checkForSensitiveWords">
<span v-for="word in sensitiveWords" :key="word" class="highlighted-word">{{ word }}</span>
</div>
</template>
```
3. **添加数据和方法**:
```js
import SensitiveWordFilter from 'vue-sensitive-word-filter';
export default {
components: {
SensitiveWordFilter,
},
data() {
return {
searchText: '',
sensitiveWords: [],
};
},
methods: {
checkForSensitiveWords(e) {
const filter = new SensitiveWordFilter(this.sensitiveWords);
this.highlightedWords = filter.filter(this.searchText);
},
},
}
```
4. **配置敏感词列表**:
将你要高亮的敏感词存储在`sensitiveWords`数组中,例如:
```js
computed: {
highlightedWords() {
return this.searchText.split(' ').filter(word => this.sensitiveWords.includes(word));
},
},
data() {
return {
...,
sensitiveWords: ['关键词1', '关键词2', '关键词3'],
};
},
```
5. **样式设置**:
添加CSS规则以高亮显示敏感词,比如:
```css
.highlighted-word {
background-color: yellow;
font-weight: bold;
}
```
阅读全文
相关推荐
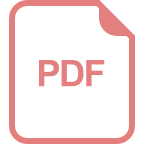
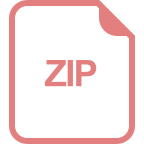
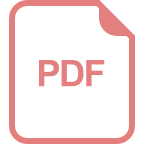
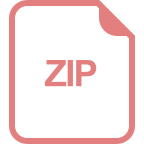
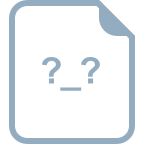
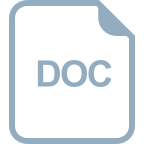
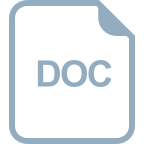
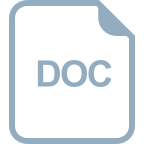
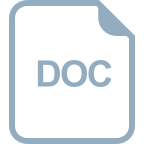





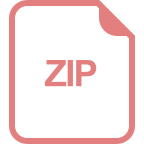