c语言设计一个算法,用于实现子串替换(在链串上实现)。
时间: 2024-11-20 20:36:18 浏览: 11
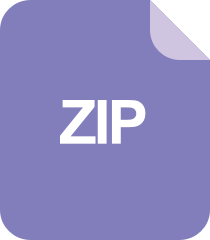
改进KMP算法.zip_KMP算法_c语言数据结构

在C语言中,我们可以使用链表数据结构来实现一个子串替换算法。这里是一个简单的步骤说明:
1. **定义链表节点**:
首先,我们需要定义一个链表节点结构,包含字符值和指向下一个节点的指针。
```c
typedef struct Node {
char data;
struct Node* next;
} ListNode;
```
2. **链表操作函数**:
- `initList`: 初始化空链表。
- `append`: 向链表末尾添加字符。
- `findSubstring`: 查找给定字符串是否是链表的一个子串。
- `replaceSubstring`: 替换子串。
```c
ListNode* initList() {
ListNode* head = NULL;
return head;
}
void append(ListNode* list, char ch) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = ch;
newNode->next = NULL;
if(list == NULL) {
list = newNode;
} else {
ListNode* temp = list;
while(temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
int findSubstring(ListNode* list, const char* subStr) {
ListNode* current = list;
int index = 0;
for(int i = 0; subStr[i] != '\0'; i++) {
while(current != NULL && current->data != subStr[i]) {
current = current->next;
}
if(current == NULL) {
break;
}
current = current->next;
index++;
}
if(current != NULL) {
return 1; // 子串存在
} else {
return 0; // 子串不存在
}
}
void replaceSubstring(ListNode* list, const char* oldSubStr, const char* newSubStr) {
if(findSubstring(list, oldSubStr)) {
int pos = 0;
while(findSubstring(list, oldSubStr, pos)) {
int len = strlen(oldSubStr);
for(int i = 0; i < len; i++) {
ListNode* temp = list;
for(int j = 0; j < i; j++) {
temp = temp->next;
}
temp->data = newSubStr[j];
}
pos += len;
}
}
}
```
3. **主函数示例**:
测试上述函数并创建链表实例。
```c
int main() {
ListNode* list = initList();
// ... 添加字符到链表 ...
char oldSubStr[] = "old";
char newSubStr[] = "new";
replaceSubstring(list, oldSubStr, newSubStr);
// 打印替换后的链表...
return 0;
}
```
阅读全文
相关推荐
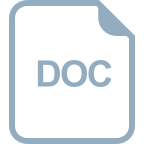
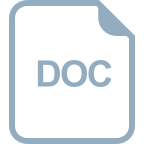


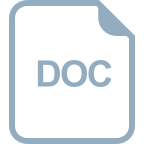












