resttemplate 忽略证书
时间: 2023-10-04 18:12:46 浏览: 55
要在使用 RestTemplate 时忽略证书,你可以创建一个自定义的 RestTemplate 实例,并配置一个自定义的 HttpClient 来处理 SSL 验证。以下是一个示例:
```java
import org.apache.http.client.HttpClient;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.client.HttpClients;
import org.springframework.http.client.HttpComponentsClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
import java.security.cert.X509Certificate;
public class CustomRestTemplate {
public static RestTemplate createRestTemplate() throws Exception {
// 创建信任所有证书的 SSLContext
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, new TrustManager[] { new X509TrustManager() {
public X509Certificate[] getAcceptedIssuers() {
return null;
}
public void checkClientTrusted(X509Certificate[] certs, String authType) {}
public void checkServerTrusted(X509Certificate[] certs, String authType) {}
}}, null);
// 创建忽略证书验证的 HttpClient
HttpClient httpClient = HttpClients.custom()
.setSSLHostnameVerifier(NoopHostnameVerifier.INSTANCE)
.setSSLContext(sslContext)
.build();
// 使用自定义的 HttpClient 创建 HttpComponentsClientHttpRequestFactory
HttpComponentsClientHttpRequestFactory requestFactory = new HttpComponentsClientHttpRequestFactory(httpClient);
// 创建 RestTemplate 实例并设置请求工厂
RestTemplate restTemplate = new RestTemplate(requestFactory);
return restTemplate;
}
public static void main(String[] args) {
try {
RestTemplate restTemplate = createRestTemplate();
// 使用忽略证书的 RestTemplate 发送请求
String response = restTemplate.getForObject("https://example.com/api", String.class);
System.out.println(response);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述示例中,我们创建了一个自定义的 RestTemplate 实例 `restTemplate`,该实例使用了一个自定义的 HttpClient,该 HttpClient 忽略了证书验证。你可以根据自己的需求修改 `createRestTemplate` 方法中的代码,并使用 `restTemplate` 对象来发送 HTTP 请求。请注意,忽略证书验证可能会带来安全风险,请慎重使用。
相关推荐
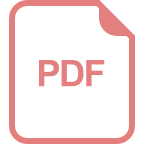
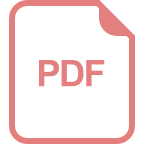














