c++replace
时间: 2023-08-26 11:17:48 浏览: 53
在C++中,你可以使用`std::string`的`replace`函数来替换字符串中的特定部分。这个函数有几个不同的重载形式,我将演示其中的一种常见用法。
下面是一个示例代码,展示了如何使用`replace`函数来替换字符串中的子串:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
std::cout << "原始字符串: " << str << std::endl;
// 使用 replace 函数替换子串
str.replace(6, 5, "C++");
std::cout << "替换后的字符串: " << str << std::endl;
return 0;
}
```
在上面的示例中,我们将字符串`"Hello World!"`中的子串`"World"`替换为`"C++"`。`replace`函数的第一个参数是要替换的子串的起始位置,第二个参数是要替换的子串的长度,第三个参数是要替换成的新字符串。
运行上述代码,输出将会是:
```
原始字符串: Hello World!
替换后的字符串: Hello C++!
```
希望这个示例能够帮助你理解如何在C++中使用`replace`函数进行字符串替换。如果你有任何进一步的问题,请随时提问!
相关问题
c++ replace
在C++中,你可以使用`std::string`类的`replace`函数来替换字符串中的特定部分。`replace`函数的用法如下:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "Original string: " << str << std::endl;
// 替换字符串中的一部分
str.replace(7, 5, "C++");
std::cout << "Replaced string: " << str << std::endl;
return 0;
}
```
以上代码将输出:
```
Original string: Hello, world!
Replaced string: Hello, C++!
```
在`replace`函数中,第一个参数是要替换的起始位置,第二个参数是要替换的字符数,第三个参数是要替换成的字符串。
c++ replace string
在给定的代码示例中,使用的是C++的string类的replace函数来替换字符串中的特定内容。replace函数有多种用法,每种用法都有不同的参数和功能。
引用中给出了replace函数的一种用法,使用重复n次的字符c来替换从指定迭代器位置开始的内容。在代码示例中,通过调用replace函数将字符'1'替换了从迭代器位置开始的3个字符。最后,使用cout输出替换后的字符串。
引用中给出了replace函数的另一种用法,用字符串str替换从起始位置pos开始长度为len的字符。在代码示例中,通过调用replace函数将第一个出现的字符'@'替换为空字符串。最后,使用cout输出替换后的字符串。
引用中给出了replace函数的第三种用法,使用char*类型的字符串s来替换从指定迭代器位置开始的字符串。在代码示例中,通过调用replace函数将char*类型的字符串"12345"替换了从迭代器位置开始的9个字符。最后,使用cout输出替换后的字符串。
总结起来,replace函数可以根据不同的参数使用不同的方法来替换字符串中的内容。您可以根据您的需求选择适合的replace函数用法来进行字符串替换操作。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
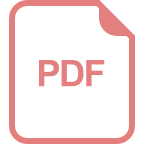
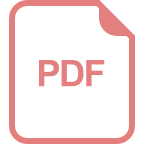
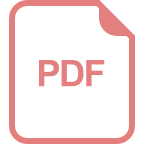












