用c语言怎么创建出二叉树的tu树形
时间: 2024-10-15 18:29:17 浏览: 21
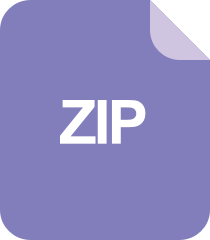
二叉树的创建与遍历C语言实现代码.zip
在C语言中创建二叉树,通常会使用结构体来表示树节点,并通过指针关联父子节点。下面是一个简单的示例,展示如何创建一个二叉搜索树(BST)的结构以及插入节点的基本操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构
typedef struct TreeNode {
int val; // 节点值
struct TreeNode* left; // 左子节点
struct TreeNode* right; // 右子节点
} TreeNode;
// 创建一个新的节点
TreeNode* createNode(int val) {
TreeNode* newNode = (TreeNode*)malloc(sizeof(TreeNode));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->val = val;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
// 插入节点到BST
TreeNode* insertNode(TreeNode* root, int val) {
if (root == NULL) { // 如果根节点为空,直接插入
return createNode(val);
}
if (val < root->val) { // 如果新值小于当前节点,向左子树递归插入
root->left = insertNode(root->left, val);
} else if (val > root->val) { // 否则向右子树递归插入
root->right = insertNode(root->right, val);
}
return root;
}
// 示例:创建一个简单的BST并插入一些节点
int main() {
TreeNode* root = NULL;
root = insertNode(root, 50); // 插入根节点
insertNode(root, 30);
insertNode(root, 70);
insertNode(root, 20);
insertNode(root, 40);
insertNode(root, 60);
// 打印树形结构(这里仅作演示,实际应用中可以自行添加函数)
printTree(root);
return 0;
}
```
阅读全文
相关推荐
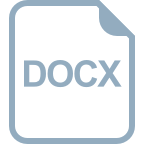
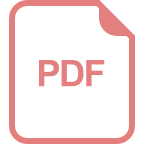
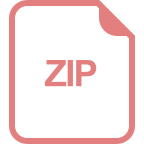
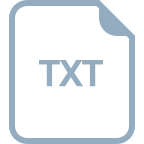
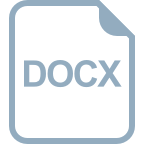
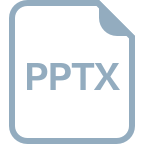
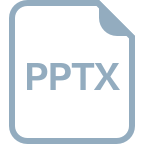
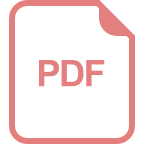
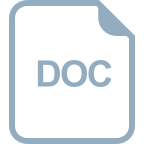
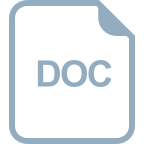


