nodejs mysql 批量插入100条数据
时间: 2025-01-04 07:40:51 浏览: 33
在Node.js中,使用`mysql2`库批量插入大量数据到MySQL数据库通常涉及预处理语句和游标(cursor),以减少网络往返次数和提高性能。以下是一个示例,演示如何批量插入100条数据:
首先,确保安装了`mysql2`模块:
```bash
npm install mysql2
```
然后,编写一个函数来进行批量插入:
```javascript
const mysql = require('mysql2/promise');
async function batchInsert(data, dbConfig) {
const connection = await mysql.createConnection(dbConfig);
const bulkInsertQuery = `INSERT INTO w_rank (id, type, info, time, valueObj, aid) VALUES ? ON DUPLICATE KEY UPDATE type=VALUES(type), info=VALUES(info), time=VALUES(time), valueObj=VALUES(valueObj), aid=VALUES(aid)`;
try {
// 创建一个预处理语句
const preparedQuery = await connection.prepare(bulkInsertQuery);
// 使用Array.from和map来生成插入值的数组
const valuesArrays = Array.from({ length: data.length }, (_, i) => data[i]);
// 使用预处理语句的batchStart和batchEnd方法分批插入数据
for (let start = 0; start < valuesArrays.length; start += 100) {
const end = Math.min(start + 100, valuesArrays.length);
await preparedQuery.batchStart();
// 分块插入数据
for (let i = start; i < end; i++) {
await preparedQuery.execute(valuesArrays[i]);
}
await preparedQuery.batchCommit(); // 执行批量提交
}
console.log('Bulk insert completed successfully.');
} catch (error) {
console.error('Error during bulk insert:', error);
} finally {
connection.end();
}
}
// 数据样例
const data = [
... // 100条数据,每条数据结构类似:[1, 'type1', 'info1', timestamp1, value1, aid1]
];
// 配置连接信息
const dbConfig = {
host: 'your_host',
user: 'your_user',
password: 'your_password',
database: 'your_database'
};
batchInsert(data, dbConfig);
```
这个示例中,我们把数据分为100条一组进行插入,每次使用`batchStart`开始一个新的批量,然后逐条插入数据,并在所有插入完成后使用`batchCommit`提交。
阅读全文
相关推荐











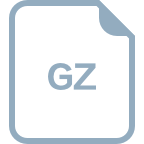





