java 代码读取其他电脑的文件
时间: 2024-12-12 07:26:11 浏览: 7
在Java中,如果你想要读取另一台计算机(通常指远程服务器或网络上的另一台机器)的文件,你需要使用网络IO技术。以下是一个简单的示例,展示如何通过Socket或FileInputStream从远程主机读取文件:
```java
import java.io.*;
import java.net.*;
public class FileTransfer {
public static void main(String[] args) throws IOException {
String remoteHost = "IP_ADDRESS";
int remotePort = 9999; // 远程服务器的端口号
String localFilePath = "/path/to/local/file.txt"; // 本地文件路径
try (ServerSocket server = new ServerSocket(remotePort);
Socket client = new Socket(remoteHost, remotePort)) {
FileInputStream fis = new FileInputStream(localFilePath); // 本地文件输入流
DataOutputStream dos = new DataOutputStream(client.getOutputStream()); // 发送数据到客户端的输出流
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
dos.write(buffer, 0, bytesRead); // 将读取的数据发送给客户端
}
fis.close();
dos.flush(); // 确保所有数据已发送完毕
System.out.println("Local file sent successfully");
} catch (IOException e) {
System.err.println("Error reading or sending file: " + e.getMessage());
}
}
}
```
在这个例子中,首先创建一个ServerSocket监听指定的端口,然后接收来自客户端的连接。接着,使用FileInputStream读取本地文件的内容,并通过DataOutputStream发送到连接的客户端。
然而,这需要目标机器已经配置了相应的服务,允许文件的访问,通常在实际应用中会涉及到权限管理和安全控制。另外,上述代码仅适用于文本文件,二进制文件的传输需稍作修改。
阅读全文
相关推荐




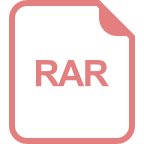



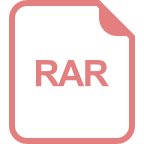

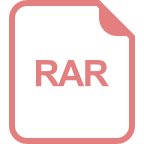







