SSD算法代码,python
时间: 2024-08-16 19:01:02 浏览: 52
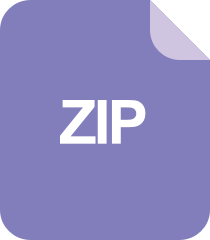
基于Pytorch实现SSD算法并进行改进python源码(本科毕业设计).zip
SSD (Single Shot Detection) 是一种广泛用于物体检测的深度学习模型,它在单次前向传播中就能完成物体的位置预测和类别识别。在Python中,可以使用TensorFlow Object Detection API来实现SSD模型。以下是一个基本的示例,展示如何加载预训练模型并进行预测:
```python
# 导入所需的库
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as viz_utils
from object_detection.utils import ops as utils_ops
# 加载预训练模型和标签映射
model_name = 'ssd_mobilenet_v1_coco_11_06_2017'
PATH_TO_CKPT = model_name + '/frozen_inference_graph.pb'
label_map_path = model_name + '/data/mscoco_label_map.pbtxt'
category_index = label_map_util.create_category_index_from_labelmap(label_map_path, use_display_name=True)
# 加载模型
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(PATH_TO_CKPT, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
sess = tf.Session(graph=detection_graph)
# 输入图像路径
image_path = 'path_to_your_image.jpg'
# 获取图像数据
image_data = Image.open(image_path).convert('RGB')
image_tensor = detection_graph.get_tensor_by_name('image_tensor:0')
# 检测操作
boxes = detection_graph.get_tensor_by_name('detection_boxes:0')
scores = detection_graph.get_tensor_by_name('detection_scores:0')
classes = detection_graph.get_tensor_by_name('detection_classes:0')
num_detections = detection_graph.get_tensor_by_name('num_detections:0')
# 进行预测
(boxes, scores, classes, num_detections) = sess.run(
[boxes, scores, classes, num_detections],
feed_dict={image_tensor: np.expand_dims(image_data, axis=0)})
# 可视化结果
vis_util.visualize_boxes_and_labels_on_image_array(
image_data,
np.squeeze(boxes),
np.squeeze(classes).astype(np.int32),
np.squeeze(scores),
category_index,
use_normalized_coordinates=True,
line_thickness=8)
# 显示图片
plt.figure(figsize=(12, 8))
plt.imshow(image_data)
plt.show()
# 关闭会话
sess.close()
```
请注意,这只是一个基础示例,实际应用可能需要对输入图像进行预处理,以及处理多张图片或实时视频流。此外,这个例子假设您已经安装了TensorFlow Object Detection API。
阅读全文
相关推荐
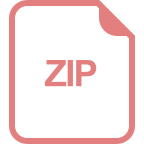
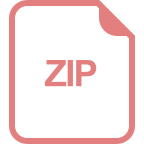
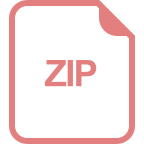
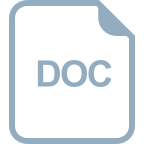
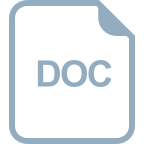


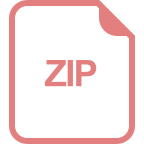
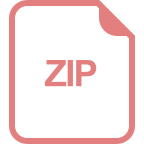
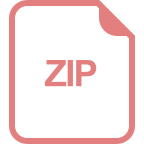
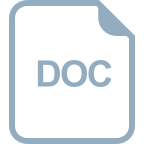
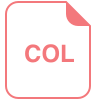
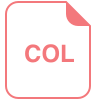
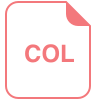
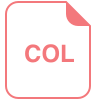


