用c语言实现上述实验要求
时间: 2024-10-12 20:05:13 浏览: 17
在C语言中实现这样的算术表达式解析器,你可以采用递归下降解析器(Recursive Descent Parser)的方法。下面是一个简单的示例,包括合法性检查和基本的求值功能:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <stdbool.h>
typedef struct ExpNode {
enum { NUMBER, OPERAND, LEFT_PAREN, RIGHT_PAREN } type;
union {
int num;
char *str;
struct ExpNode *left, *right;
} data;
} ExpNode;
ExpNode *parse_exp(const char *input);
int eval_exp(ExpNode *node);
bool is_number(char c) {
return (isdigit(c) || c == '#');
}
ExpNode *number_or_op(char c) {
if (is_number(c)) {
return create_node(NUMBER, (union { int; char* })c - '0');
}
else if (c == '+' || c == '-' || c == '*' || c == '/') {
return create_node(OPERAND, (union { int; char* })c);
}
else if (c == '(') {
return create_node(LEFT_PAREN, NULL);
}
else if (c == ')') {
return create_node(RIGHT_PAREN, NULL);
}
return NULL;
}
ExpNode *parse_exp(const char *input) {
ExpNode *node = number_or_op(*input);
while (*input != '\0') {
if (node->type == LEFT_PAREN && node->data.left == NULL) {
node->data.left = parse_exp(input + 1);
input++;
}
else if (node->type == OPERAND && input[1] != '\0' && is_number(input[1])) {
// 括号内的数值直接连接
node->data.left = parse_exp(input + 1);
}
else {
break;
}
node = number_or_op(input[0]);
if (node->type == RIGHT_PAREN && node->data.left == NULL) {
break;
}
}
return node;
}
int eval_exp(ExpNode *node) {
switch (node->type) {
case NUMBER:
return node->data.num;
case OPERAND:
// 实际运算需要根据优先级规则进一步处理
return node->data.num;
case LEFT_PAREN:
return eval_exp(node->data.left);
case RIGHT_PAREN:
return 0;
}
}
int main() {
char exp[] = "3 # + 5 * ( 2 / 1 )";
ExpNode *root = parse_exp(exp);
if (root->type == RIGHT_PAREN) {
printf("Expression is invalid.\n");
free(root);
return 1;
}
int result = eval_exp(root);
printf("Result: %d\n", result);
free(root);
return 0;
}
```
注意,这个示例只是一个基础版本,实际的实现还需要考虑更复杂的操作符优先级规则以及错误处理。此外,对于输入的完整合法性检查(如平衡括号和完整表达式等)可能需要额外的辅助函数。
阅读全文
相关推荐
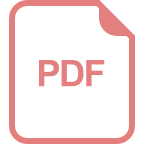
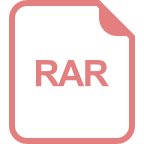
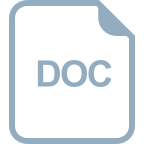


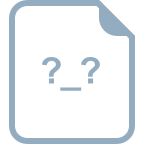
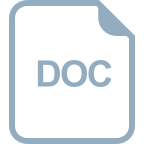
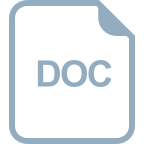
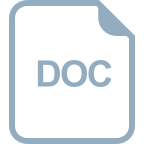
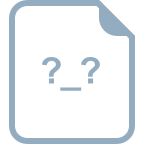
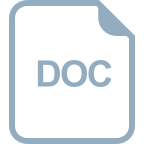
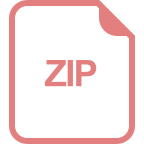
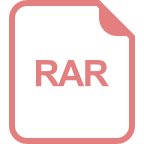
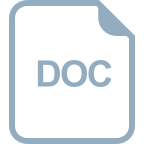
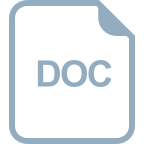
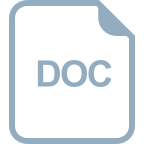
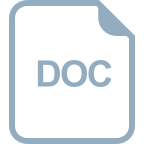
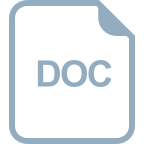
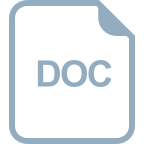