react hooks useTable
时间: 2023-11-08 10:55:18 浏览: 50
在 React Hooks 中,并没有内置的 useTable 方法。但是,你可以使用一些第三方库,如 react-table 或者 react-bootstrap-table-next,来实现一个功能强大的表格组件。
举例来说,如果你想使用 react-table,你可以按照以下步骤进行安装和使用:
1. 安装 react-table:
```bash
npm install react-table
```
2. 导入所需的组件和样式:
```jsx
import React from "react";
import { useTable } from "react-table";
import "react-table/react-table.css";
```
3. 创建一个自定义的表格组件,并使用 useTable Hook 来处理数据和列的配置:
```jsx
function MyTable({ columns, data }) {
const {
getTableProps,
getTableBodyProps,
headerGroups,
rows,
prepareRow
} = useTable({
columns,
data
});
return (
<table {...getTableProps()} className="table">
<thead>
{headerGroups.map(headerGroup => (
<tr {...headerGroup.getHeaderGroupProps()}>
{headerGroup.headers.map(column => (
<th {...column.getHeaderProps()}>{column.render("Header")}</th>
))}
</tr>
))}
</thead>
<tbody {...getTableBodyProps()}>
{rows.map(row => {
prepareRow(row);
return (
<tr {...row.getRowProps()}>
{row.cells.map(cell => (
<td {...cell.getCellProps()}>{cell.render("Cell")}</td>
))}
</tr>
);
})}
</tbody>
</table>
);
}
```
4. 在你的组件中使用 MyTable 组件,并传递列和数据的配置:
```jsx
function App() {
const columns = [
{ Header: "Name", accessor: "name" },
{ Header: "Age", accessor: "age" },
{ Header: "Gender", accessor: "gender" }
];
const data = [
{ name: "John Doe", age: 25, gender: "Male" },
{ name: "Jane Smith", age: 30, gender: "Female" },
{ name: "Bob Johnson", age: 40, gender: "Male" }
];
return (
<div className="App">
<MyTable columns={columns} data={data} />
</div>
);
}
```
在上述代码中,我们创建了一个自定义的表格组件 MyTable,并使用 useTable Hook 来处理列和数据的配置。我们使用了 react-table 提供的一些方法和属性来渲染表格的头部和主体部分。
这样,你就可以使用 react-table 和 useTable Hook 来创建一个功能完善的表格组件。同样的,你也可以根据你的需求选择其他类似的第三方库来实现表格功能。
相关推荐
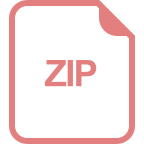
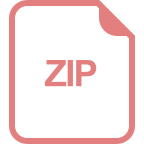













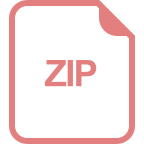
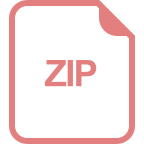