C# 删除指定路径下过期的文件夹
时间: 2024-10-13 22:07:49 浏览: 30
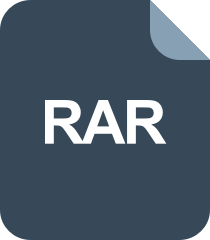
C#定时删除带日期的文件
在C#中,删除指定路径下的过期文件夹通常涉及到文件系统操作,特别是当需要检查文件的时间戳是否超过某个设定的过期时间。这里有一个基本的示例,展示如何使用`Directory.Delete()`方法配合`DateTime`类来实现这个功能:
```csharp
using System;
using System.IO;
public static bool DeleteExpiredFolders(string folderPath, DateTime expirationDate)
{
// 检查路径是否存在且是文件夹
if (!Directory.Exists(folderPath))
{
Console.WriteLine($"The specified path {folderPath} does not exist or is not a folder.");
return false;
}
try
{
// 遍历文件夹中的所有文件和子文件夹
foreach (string fileOrFolder in Directory.GetFiles(folderPath).Concat(Directory.GetDirectories(folderPath)))
{
// 获取文件或目录的最后修改日期
DateTime lastModified = File.GetLastWriteTimeUtc(fileOrFolder);
// 如果已过期
if (lastModified < expirationDate)
{
// 删除文件
if (File.Exists(fileOrFolder))
File.Delete(fileOrFolder);
// 或者递归删除子文件夹
else if (Directory.Exists(fileOrFolder))
Directory.Delete(fileOrFolder, true); // 第二个参数设为true表示删除空的文件夹
}
}
Console.WriteLine($"Deleted all expired files and folders from {folderPath}.");
return true;
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred while deleting: {ex.Message}");
return false;
}
}
// 使用示例
DateTime oneMonthAgo = DateTime.UtcNow.AddMonths(-1);
bool result = DeleteExpiredFolders(@"C:\path\to\check", oneMonthAgo);
```
在这个例子中,你需要替换`@C:\path\to\check`为你想要检查的实际文件夹路径,并提供一个过期日期,如一个月前。
阅读全文
相关推荐
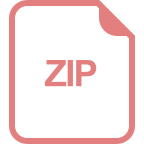
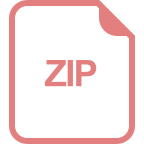




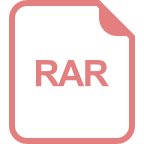
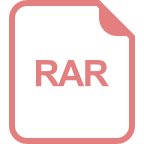
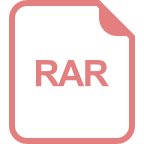
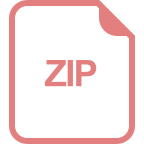
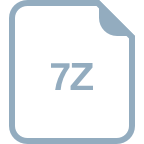
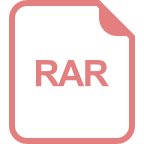
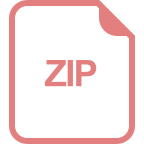
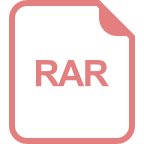
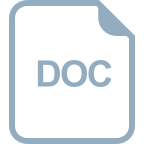
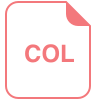
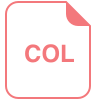
