c++交统计单词个数
时间: 2024-04-14 21:22:32 浏览: 28
在C++中,统计单词个数可以通过以下步骤实现:
1. 读取文本文件:首先,你需要打开并读取包含文本的文件。你可以使用`ifstream`类来打开文件,并使用`getline`函数逐行读取文本内容。
2. 分割单词:对于每一行的文本内容,你可以使用字符串流(`stringstream`)将其分割成单词。可以使用空格或标点符号作为分隔符,将每个单词提取出来。
3. 统计单词个数:使用一个计数器变量,每当提取到一个单词时,计数器加一。
4. 输出结果:最后,输出统计得到的单词个数。
下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
int countWords(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
std::cout << "Failed to open the file." << std::endl;
return 0;
}
std::string line;
int wordCount = 0;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string word;
while (ss >> word) {
wordCount++;
}
}
file.close();
return wordCount;
}
int main() {
std::string filename = "example.txt";
int wordCount = countWords(filename);
std::cout << "Total number of words: " << wordCount << std::endl;
return 0;
}
```
请注意,上述示例代码假设你已经创建了一个名为`example.txt`的文本文件,并将其放置在与代码文件相同的目录下。你可以根据实际情况修改文件名和路径。
相关推荐
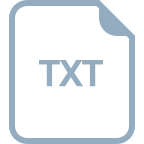














