编写一个C++程序用链表实现输入通讯公司名称,查询使用该公司手机同学的信息。
时间: 2024-09-24 13:02:00 浏览: 30
在C++中,我们可以创建一个简单的链表结构来存储学生的数据,包括姓名、电话号码以及所属的通讯公司。下面是一个简化的示例程序,演示了如何实现这个功能:
```cpp
#include <iostream>
#include <string>
// 定义链表节点
struct Student {
std::string name;
std::string phone;
std::string company;
// 指针成员用于链接下一个节点
struct Student* next;
};
// 添加学生信息到链表
void addStudent(Student** head, const std::string& name, const std::string& phone, const std::string& company) {
Student* newNode = new Student();
newNode->name = name;
newNode->phone = phone;
newNode->company = company;
newNode->next = nullptr;
if (*head == nullptr) {
*head = newNode;
} else {
Student* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 查询使用指定公司手机的同学
void searchCompany(const Student* head, const std::string& company) {
if (head == nullptr) {
std::cout << "No students found.\n";
return;
}
std::cout << "Students using " << company << " phones:\n";
Student* current = head;
while (current != nullptr) {
if (current->company == company) {
std::cout << "Name: " << current->name << ", Phone: " << current->phone << "\n";
}
current = current->next;
}
}
int main() {
Student* head = nullptr; // 初始化链表头部
// 添加一些学生信息
addStudent(&head, "张三", "13800138000", "移动");
addStudent(&head, "李四", "13900139000", "电信");
addStudent(&head, "王五", "15000150000", "联通");
// 查询使用移动公司的学生
searchCompany(head, "移动");
return 0;
}
```
在这个程序里,用户可以添加学生信息并随时通过`searchCompany`函数查询特定公司的学生。当询问一个不存在的公司时,会提示没有找到相关的学生。
阅读全文
相关推荐
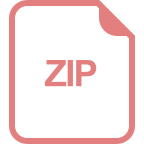
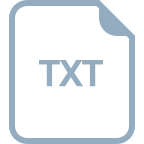
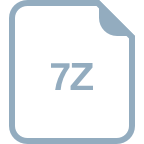
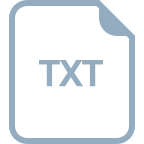
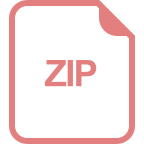
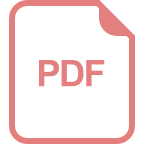
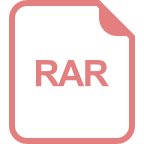
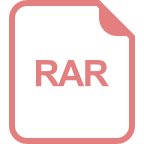
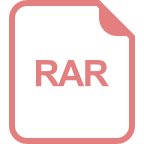
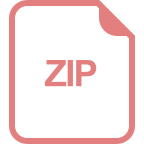
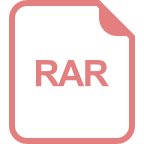
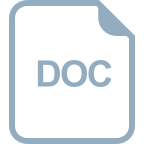