遗传算法多式联运python
时间: 2024-07-05 12:01:12 浏览: 79
遗传算法(Genetic Algorithm, GA)是一种模拟自然选择和遗传机制的优化搜索方法,常用于解决复杂问题的全局最优解。在Python中,有许多库可以帮助我们实现遗传算法,如`deap`(Distributed Evolutionary Algorithms in Python)是一个流行的选择。
多目标遗传算法(Multi-objective Genetic Algorithm, MOGA)则是针对涉及多个优化目标的问题,它试图找到一系列被称为Pareto最优解的解决方案集合,每个解决方案都不劣于其他任何解决方案,但在某些目标上不优于它们。
以下是使用`deap`实现遗传算法多目标优化的基本步骤:
1. **定义个体**(Individual):创建一个包含多个目标值的个体,通常用列表或元组表示。
2. **编码**(Encoding):确定如何将问题的解决方案转化为基因串,比如二进制编码、实数编码等。
3. **适应度函数**(Fitness Function):计算每个个体对所有目标的评价,多目标问题通常采用非负的Pareto效率指标。
4. **选择操作**(Selection):选择概率较高的个体,常用的方法有轮盘赌选择、 Tournament 选择等。
5. **交叉**(Crossover):结合两个父代个体的部分基因,生成新的子代。
6. **变异**(Mutation):随机改变个体的基因,引入多样性。
7. **迭代**(Iteration):重复上述步骤,直到达到预设的停止条件(如最大迭代次数或收敛标准)。
```python
from deap import base, creator, tools
# 初始化
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10.0, 10.0)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=problem_size)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 基本操作
toolbox.register("evaluate", evaluate_fitness, objectives=objective_functions) # 自定义适应度函数
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=1, indpb=0.2)
toolbox.register("select", tools.selTournament, tournsize=3)
# 进行遗传算法
pop = toolbox.population(n=n_individuals)
for gen in range(max_generations):
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
# Crossover and mutation
offspring = toolbox.mate(offspring, offspring) # 示例:两代交叉
offspring = [toolbox.mutate(ind) for ind in offspring]
# Evaluate the fitness of the offspring
fitness_values = toolbox.map(toolbox.evaluate, offspring)
# Update the population
pop[:] = offspring
pop, logbook = tools.selBest(pop, len(pop)), tools.Logbook()
logbook.record(gen=gen, evals=len(pop), fitness_max=pop.fitness.values)
print(logbook.stream, end="\n\n")
```
阅读全文
相关推荐
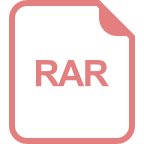


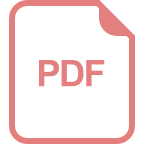
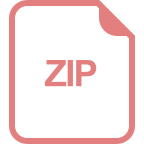
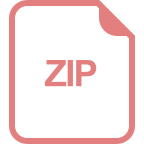
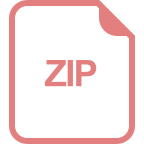
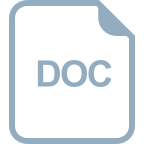
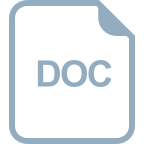
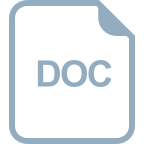
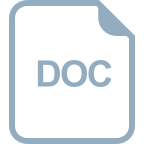




